Web API Controller
Last Updated on July 05, 2022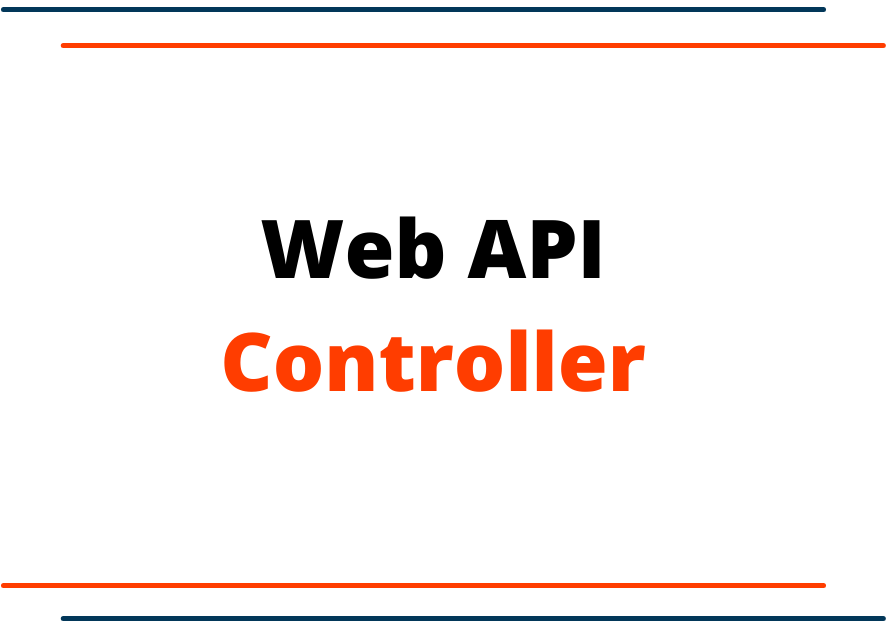
Web API Controller
Somehow, we must handle the incoming HTTP request and the API controller helps to do that job for us. It is basically like the MVC controller which can be created under the controller folder. The controller must end with “xxxxxController” and need to inherit from ‘using Microsoft.AspNetCore.Mvc.ControllerBase’.
Let’s see some examples of Web API Controller and how to create and use it.
- Create the new controller with “demoAPIController.cs” under the Controller folders.
- Add some action methods and, add the route as well same as we have done on the demoAPIController.cs file.
- After doing the above steps then try to call the method using the web browser to see the results.
Please see the below screenshot how it is going to look like after adding the demoAPIContrller.cs
Here is the code for demoAPIController.cs file.
using Microsoft.AspNetCore.Mvc;
namespace DemoApiProject.Controllers
{
public class DemoAPIController : ControllerBase
{
[Route("greeting")]
public string WelcomeMsg()
{
return "Welcome to API course!";
}
[Route("greeting/{UserName}")]
public string WelcomeMsg(string username)
{
return "Hello - " + username + "\nWelcome to API course!";
}
[Route("Search")]
public IEnumerable
Let's call one of the examples of how to call the method which we have defined inside of the DemoApi controller. For example, let's call the WelcomeMst() method by navigating to the greeting route: https://localhost:7079/greeting.
1 Welcome to API course!
Now, let's see another example of passing the value. For example, let's call the WelcomeMsg(string username) method by navigating to the greeting route: https://localhost:7079/greeting/Someone.
1 Hello - Someone Welcome to API course!
There is one more method we have defined in DemoApi Controller. Try to call that method and see what will come as a result.