C# Hashtable
Last Updated on June 20, 2022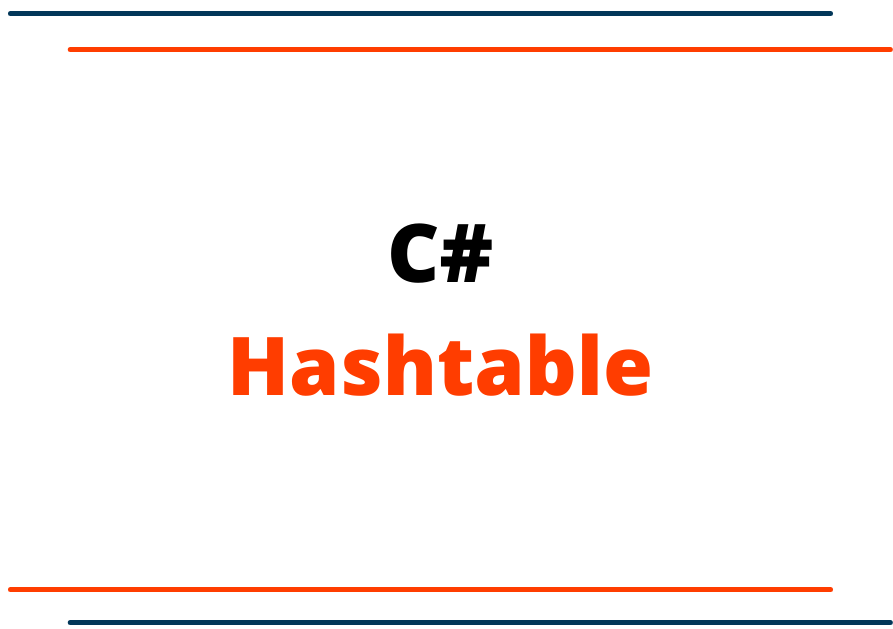
C# Hashtable
In C# the Hashtable is a non-generic collection that stores key-value pairs. It is available under System. Collections namespace. It is like the Dictionary collection. All the keys and values are object data types. Hashtable also cannot have a duplicate key, but the values can be duplicated.
Let’s see the example code of Hashtable in C#.
using System;
using System.Collections;
using System.Collections.Generic;
namespace ConsoleAppDemo
{
class Program
{
static void Main(string[] args)
{
Hashtable someData = new Hashtable();
someData.Add(1, "One");
someData.Add(2, "Two");
someData.Add(3, "Three");
someData.Add(4, "Four");
Console.WriteLine(someData[1]); //Output: One
//Access the Hashtable collection values
foreach (DictionaryEntry item in someData)
{
Console.WriteLine(item.Key + " " + item.Value);
}
Console.ReadKey();
}
}
}
Output:
4 Four 3 Three 2 Two 1 One
Let's see with another example of Hashtable using collection-initializer syntax.
using System;
using System.Collections;
using System.Collections.Generic;
namespace ConsoleAppDemo
{
class Program
{
static void Main(string[] args)
{
var someCities = new Hashtable() {
{"USA", "Kentucky, New York, Chicago"},
{"UK", "London, Manchester, Birmingham"},
{"Nepal", "Kathmandu, Pokhara, Dhulikhel"}
};
Console.WriteLine(someCities["Nepal"]); //Output: Kathmandu, Pokhara, Dhulikhel
//Access the Hashtable collection values
foreach (DictionaryEntry item in someCities)
{
Console.WriteLine(item.Key + " " + item.Value);
}
Console.ReadKey();
}
}
}
Output:
Key = Nepal, Value = Kathmandu, Pokhara, Dhulikhel Key = USA, Value = Kentucky, New York, Chicago Key = UK, Value = London, Manchester, Birmingham