C# Lists
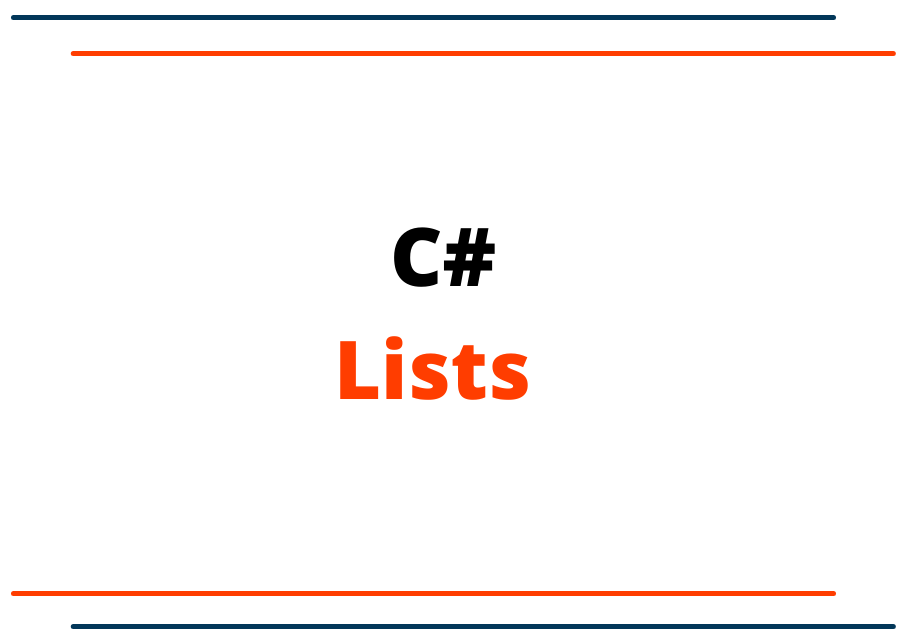
C# Lists
In C# List is one of the generic collection classes that are present under System.Collections.Generic namespace. A list is used to create a collection of any data type. The object that is stored in the List, can be accessed by its index position just like a normal array. But List can grow automatically.
Let’s look at some examples of the List in C#.
using System;
using System.Collections.Generic; //Need to add the namespace
namespace ConsoleAppDemo
{
class Program
{
static void Main(string[] args)
{
var someRandomNums = new List();
someRandomNums.Add(5);
someRandomNums.Add(10);
someRandomNums.Add(15);
someRandomNums.Add(20);
//One way to access the value of the List collection
Console.WriteLine(someRandomNums[0]); //Output: 5
//Seond way to access the value of the List collection using the loops
foreach (var nums in someRandomNums)
{
Console.WriteLine(nums);
}
//Third way to access the value of the List collection using the for loops
for (int i = 0; i < someRandomNums.Count; i++)
{
Console.WriteLine(someRandomNums[i]);
}
Console.ReadKey();
}
}
}
The output of the both the Foreach and For loops:
5 10 15 20
Let's see another example with using collection initializer syntax.
using System;
using System.Collections.Generic;
namespace ConsoleAppDemo
{
class Program
{
static void Main(string[] args)
{
var programmingLangsLists = new List()
{
"C#",
"JavaScript",
"Java",
"Python"
};
//One way to access the value of the List collection
Console.WriteLine(programmingLangsLists[0]); //Output: C#
//Seond way to access the value of the List collection using the loops
foreach (var lang in programmingLangsLists)
{
Console.WriteLine(lang);
}
//Third way to access the value of the List collection using the for loops
for (int i = 0; i < programmingLangsLists.Count; i++)
{
Console.WriteLine(programmingLangsLists[i]);
}
Console.ReadKey();
}
}
}
The output of the both the Foreach and For loops:
C# JavaScript Java Python
Let's see another example of using the class. We have created the Languages Class for the example.
using System;
using System.Collections.Generic;
namespace ConsoleAppDemo
{
//Languages Class
class Languages
{
public int Id { get; set; }
public string Name { get; set; }
}
class Program
{
static void Main(string[] args)
{
var langsLists = new List()
{
new Languages(){ Id=1, Name="C#" },
new Languages(){ Id=2, Name="JavaScript" },
new Languages(){ Id=3, Name="Java" },
new Languages(){ Id=4, Name="Python" }
};
//One way to access the value of the List collection
Console.WriteLine(langsLists[0].Id + ":" +langsLists[0].Name); //Output: 1:C#
//Seond way to access the value of the List collection using the loops
foreach (var lang in langsLists)
{
Console.WriteLine(lang.Id + ":" + lang.Name);
}
//Third way to access the value of the List collection using the for loops
for (int i = 0; i < langsLists.Count; i++)
{
Console.WriteLine(langsLists[i].Id + ":" + langsLists[i].Name);
}
Console.ReadKey();
}
}
}
The output of the both the Foreach and For loops:
1:C# 2:JavaScript 3:Java 4:Python