C# Stack
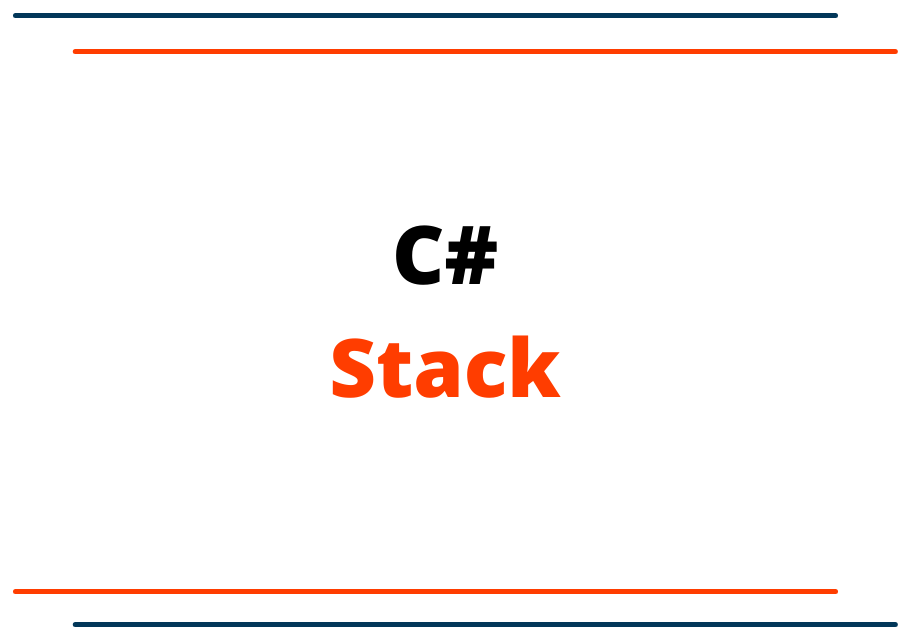
C# Stack
In C# the Stack collection is the special type of collection that stores elements in LIFO (Last in First out) style. The Stack collection is available in both generic and non-generic types of collection classes so it is present in both System.Collection.Generic and System.Collection namespace.
Let’s see the simple code example of the generic Stack collection.
using System;
using System.Collections;
using System.Collections.Generic;
namespace ConsoleAppDemo
{
class Program
{
static void Main(string[] args)
{
Stack stackDemo = new Stack();
stackDemo.Push(1);
stackDemo.Push(2);
stackDemo.Push(3);
stackDemo.Push(4);
foreach (var item in stackDemo)
{
Console.Write(item + "\n");
}
Console.ReadKey();
}
}
}
Output:
4 3 2 1
We can also use the non-generic type of Stack collection to add any types of elements.
using System;
using System.Collections;
using System.Collections.Generic;
namespace ConsoleAppDemo
{
class Program
{
static void Main(string[] args)
{
Stack stackDemo = new Stack();
stackDemo.Push("One");
stackDemo.Push(2);
stackDemo.Push("Josh");
stackDemo.Push("C");
foreach (var item in stackDemo)
{
Console.Write(item + "\n");
}
Console.ReadKey();
}
}
}
Output:
C Josh 2 One