C# SortedList
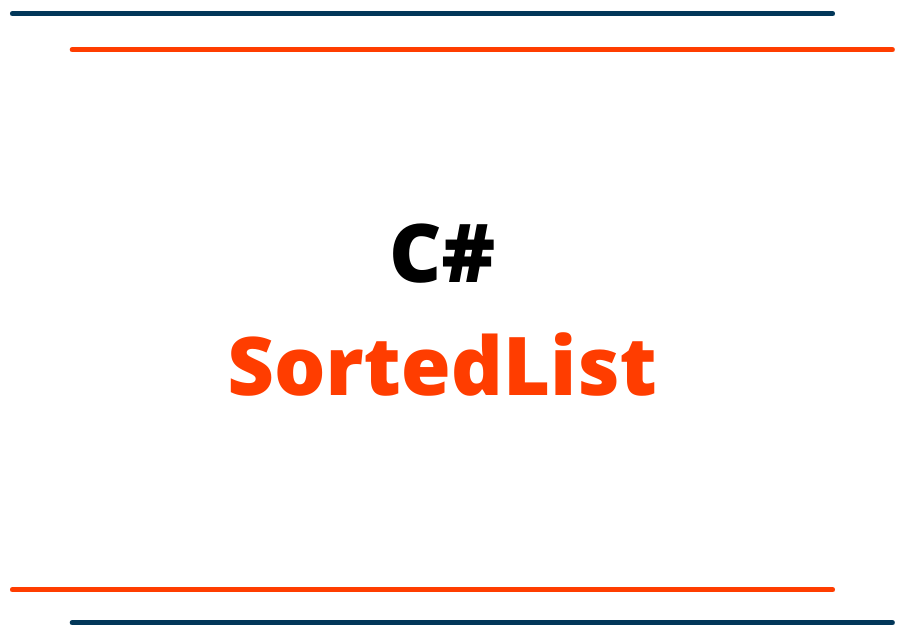
C# SortedList
In C# the SortedList collection stores key-value pairs in ascending order of the key. C# can support both generic and non-generic SortedList collections. We always recommended using the generic type of collection to perform the program faster and less error-prone than the non-generic type of SortedList.
Let’s see some examples of SortedList in C#.
The output of both the Foreach and For loops:
1 C# 2 JavaScript 3 Java 4 Python
Alright, let's see another example with collection-initializer syntax.
The output of both the Foreach and For loops:
1 C# 2 JavaScript 3 Java 4 Python