C# ArrayLists
Last Updated on June 15, 2022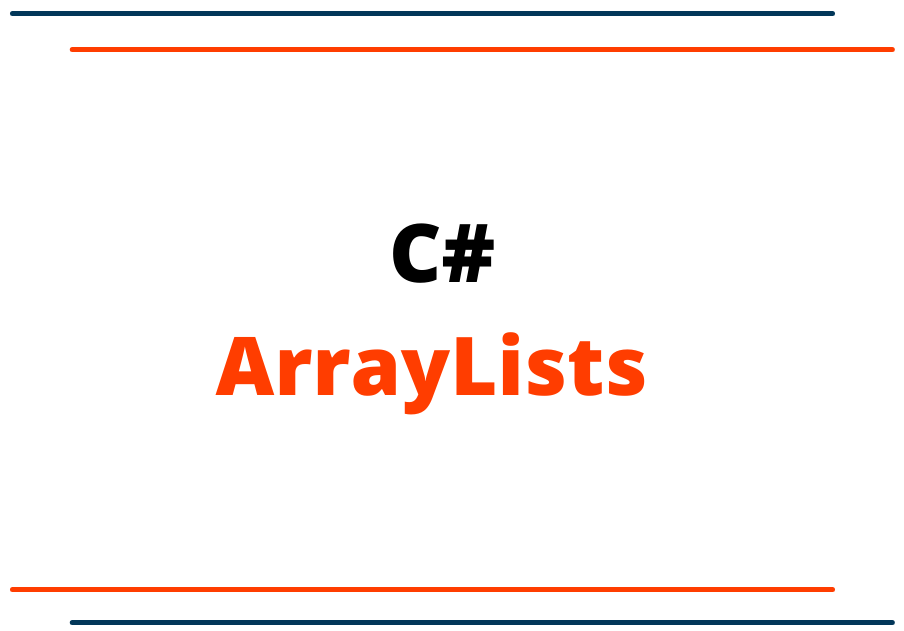
C# ArrayLists
ArrayLists is the type of the non-generic collection in C#. It can contain elements of any data type and it is similar to an array except that it can grow automatically.
Let’s see how to create an ArrayLists in C#.
using System;
using System.Collections; //Add this namespace
namespace ConsoleAppDemo
{
class Program
{
static void Main(string[] args)
{
ArrayList progLangsArr = new ArrayList(); //Don't forget to add the using System.Collections namespace
progLangsArr.Add(1);
progLangsArr.Add("Billy");
progLangsArr.Add("C");
progLangsArr.Add(true);
progLangsArr.Add(5.6);
progLangsArr.Add(null);
//Iterate an ArrayList
foreach (var item in progLangsArr)
{
Console.WriteLine(item);
}
Console.ReadKey();
}
}
}
Output:
1 Billy C True 5.6
Let's see another example with object initializer syntax.
using System;
using System.Collections;
namespace ConsoleAppDemo
{
class Program
{
static void Main(string[] args)
{
var progLangsArr = new ArrayList()
{
1, "Billy", "C", true, 5.6, null
};
//Iterate an ArrayList
foreach (var item in progLangsArr)
{
Console.WriteLine(item);
}
Console.ReadKey();
}
}
}
The result would be the same for both above examples.