C# Queue
Last Updated on June 20, 2022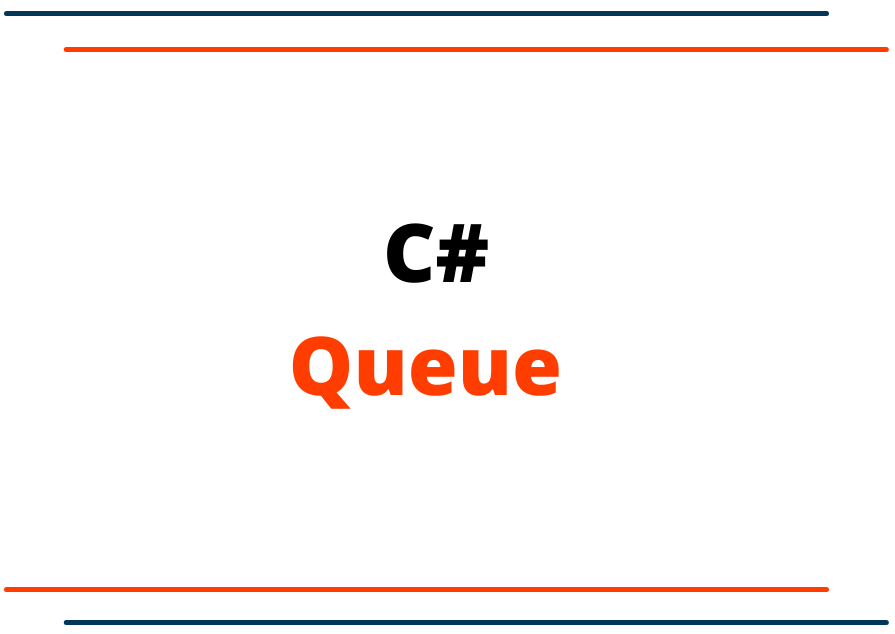
C# Queue
In C# the Queue collection is the special type of collection that stores elements in FIFO (First in First out) style. The Queue collection is available in both generic and non-generic types of collection classes so it is present in both System.Collection.Generic and System.Collection namespace.
Let’s see the simple code example of the generic Queue collection.
using System;
using System.Collections;
using System.Collections.Generic;
namespace ConsoleAppDemo
{
class Program
{
static void Main(string[] args)
{
Queue queueDemo = new Queue();
queueDemo.Enqueue(1);
queueDemo.Enqueue(2);
queueDemo.Enqueue(3);
queueDemo.Enqueue(4);
queueDemo.Enqueue(5);
foreach (var item in queueDemo)
{
Console.WriteLine(item);
}
Console.ReadKey();
}
}
}
Output:
1 2 3 4 5
Let's see another example of the non-generic type of Queue collection.
using System;
using System.Collections;
using System.Collections.Generic;
namespace ConsoleAppDemo
{
class Program
{
static void Main(string[] args)
{
Queue queueDemo = new Queue();
queueDemo.Enqueue("One");
queueDemo.Enqueue(2);
queueDemo.Enqueue("Josh");
queueDemo.Enqueue(4);
queueDemo.Enqueue("C");
foreach (var item in queueDemo)
{
Console.WriteLine(item);
}
Console.ReadKey();
}
}
}
Output:
One 2 Josh 4 C