C# Dictionary
Last Updated on June 18, 2022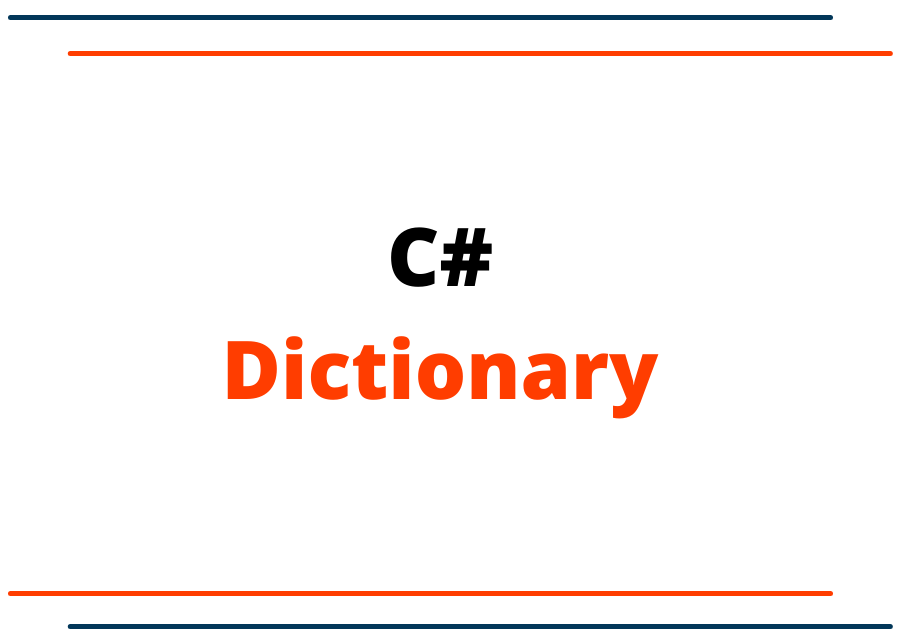
C# Dictionary
In C# the Dictionary is also another type of generic collection that stores key-value pairs. Dictionary also comes under System.Collections.Generic namespace. In a Dictionary collection key must be unique and cannot be null but values can be null or duplicated.
Let’s look at some code examples of Dictionary in C#.
using System;
using System.Collections.Generic;
namespace ConsoleAppDemo
{
class Program
{
static void Main(string[] args)
{
IDictionary<int, string> listOfEmployees = new Dictionary<int, string>();
listOfEmployees.Add(1, "Gwyn");
listOfEmployees.Add(2, "Joe");
listOfEmployees.Add(3, "Lora");
listOfEmployees.Add(4, "Nora");
listOfEmployees.Add(5, "Peter");
//Or, we can also create the Dictionary with another way
/*
var listOfEmployees = new Dictionary<int, string>()
{
{ 1, "Gwyn" },
{ 2, "Joe" },
{ 3, "Lora" },
{ 4, "Nora" },
{ 5, "Peter" },
};
*/
//One way to access the value of the Dictionary collection
Console.WriteLine(listOfEmployees[1]); //Output: Gwyn
//Seond way to access the value of the Dictionary collection using the loops
foreach (KeyValuePair<int, string> employee in listOfEmployees)
{
Console.WriteLine(employee.Value);
}
//Third way to access the value of the Dictionary collection using the for loops
for (int i = 1; i < listOfEmployees.Count; i++)
{
Console.WriteLine(listOfEmployees[i]);
}
Console.ReadKey();
}
}
}
The output of both the Foreach and For loops:
Gwyn Joe Lora Nora Peter
Let's see another example with Employee class.
using System;
using System.Collections.Generic;
namespace ConsoleAppDemo
{
class Program
{
static void Main(string[] args)
{
//Store some dummy data as an array
Employee[] listOfEmployees = {
new Employee(1, "Gwyn", 50, 45),
new Employee(2, "Joe", 35, 30),
new Employee(3, "Lora", 25, 25),
new Employee(4, "Nora", 60, 50),
new Employee(5, "Peter", 75, 55)
};
//One way to access the value of the Dictionary collection
Console.WriteLine(listOfEmployees[1].Name + " " + listOfEmployees[1].Salary); //Output: Gwyn 86400
//Seond way to access the value of the Dictionary collection using the loops
foreach (var employee in listOfEmployees)
{
Console.WriteLine(employee.Name + " " + employee.Salary);
}
//Third way to access the value of the Dictionary collection using the for loops
for (int i = 0; i < listOfEmployees.Length; i++)
{
Console.WriteLine(listOfEmployees[i].Name + " " + listOfEmployees[i].Salary);
}
Console.ReadKey();
}
}
class Employee
{
public int Id { get; set; }
public string Name { get; set; }
public int Age { get; set; }
public float Rate { get; set; }
public float Salary {
get {
return Rate * 8 * 5 * 4 * 12;
}
}
public Employee(int id, string name, int age, float rate)
{
this.Id = id;
this.Name = name;
this.Age = age;
this.Rate = rate;
}
}
}
The output of both the Foreach and For loops:
Gwyn 86400 Joe 57600 Lora 48000 Nora 96000 Peter 105600