Variable in JavaScript
Last Updated on May 03, 2022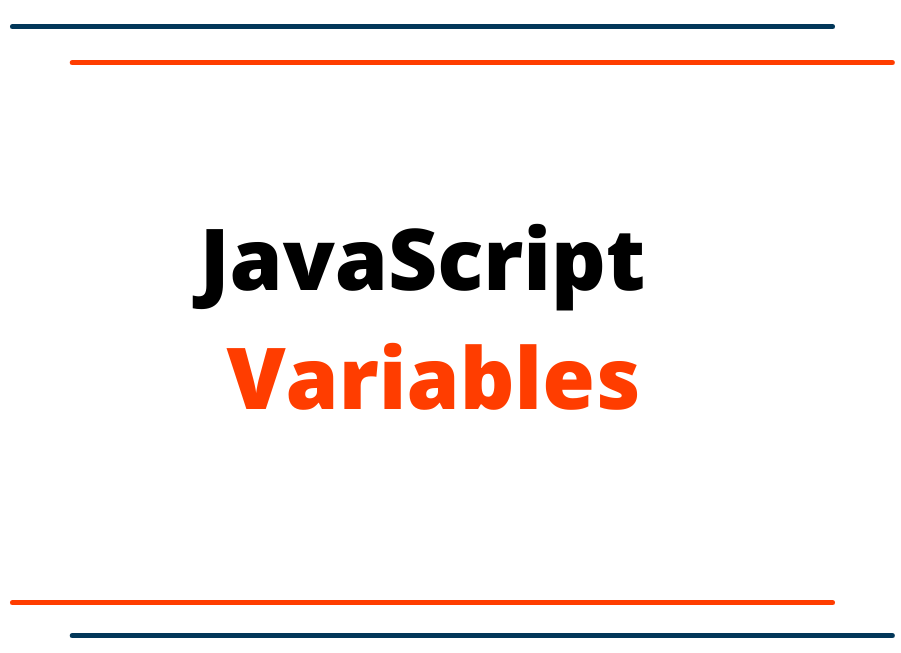
What are variables in JavaScript?
A variable is a container that holds data. It can be used to store numbers, characters, or even other variables.
Variables are one of the most fundamental concepts in programming languages. Variables can hold any type of data.
There are various ways to declare the variable in JavaScript.
- Var - Using the var keyword is one of the common ways to declare the variable in JavaScript. This keyword is supported by all kinds of web browsers. It can be redeclared.
- Let – Let keyword was introduced from ES6 (2015). Let keyword variable cannot be redeclared.
- Const – Const keyword also was introduced from ES6 (2015). Const keyword variable also cannot be redeclared.
JavaScript variables example.
// Example of using Var keyword
var x = 5;
var y = 10;
var z = x + y; //15
var z = x * y; //50
In the above code example, you can see that var z has been redeclared.
// Example of using Let keyword
let x = 5;
let y = 10;
let z = x + y; //15
let zz = x * y; //50
//It can be declared without initialization
let a;
a = "JavaScript"; //you can initialization the value
In the above code example, you can see that let z cannot be redeclared if we use the let keyword. That's why we have to declare another variable with a different name like zz.
// Example of using Const keyword
const x = 5;
const y = 10;
const z = x + y; //15
const zz = x * y; //50
//It cannot be declared without initialization
const a;
a = "JavaScript"; //Error
//const keyword must have initialized the value and cannot be redeclared
const b = "Must have to initialize some kinds of value";
In the above code example, you can see that const cannot be redeclared and must have initialized the value.