JavaScript Object
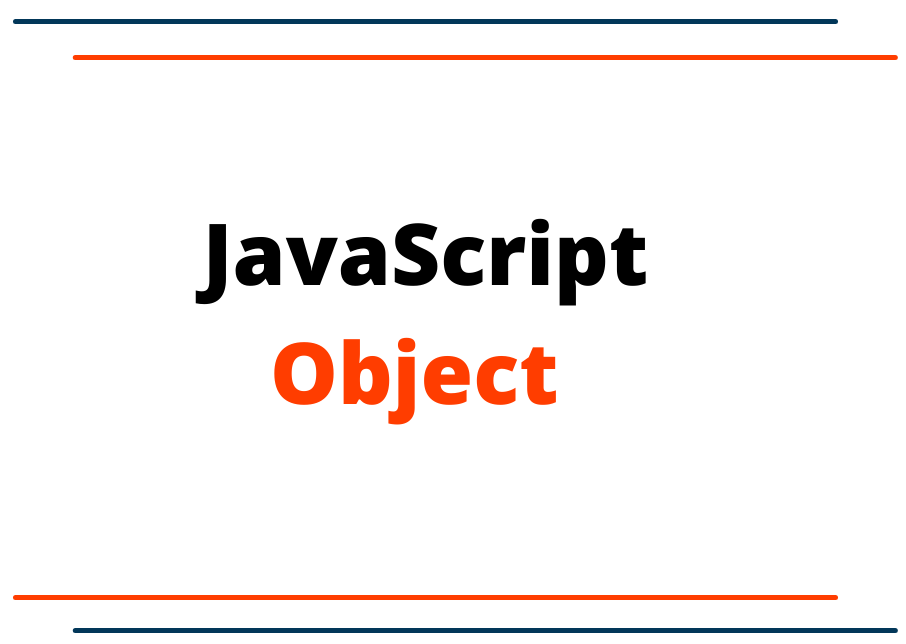
JavaScript Object
In real life, the JavaScript object is an entity that has properties and methods. For example - computers, cars, etc. JavaScript objects are data structures that can contain different types of values.
Objects are a way to group together similar values and give them a name. Objects can contain other objects and arrays. Objects can also be nested inside one another, which allows you to create complex data structures.
Let's take a look at the JavaScript object syntax and the code example of how to create an object.
NameOfObject={property1:value1,property2:value2};
// We can have many properties and values
var car = {type:"Toyota", model:"Highlander", color:"blue"};
//Or we can have line break for each properties and values for better looks of code and easy to read.
var car = {
type: "Toyota",
model: "Highlander",
color: "blue"
};
Another way to create an object with using new keyword in JavaScript
var car = new Object();
car.type = "Toyota";
car.model = "Highlander";
car.color = "blue";
Objects could also have the methods which can store as an object properties. A method is a function that can stored as a property of the object.
var car = {
type: "Toyota",
model: "Highlander",
color: "blue",
getCarDetails: function(){
return this.type + " " + this.model + " " + this.color;
}
};
this keyword refers to the car object which means this refers to the current object
We will show you more examples later but for now, let's try to access the object property and value
var car = {
type: "Toyota",
model: "Highlander",
color: "blue",
getCarDetails: function(){
return this.type + " " + this.model + " " + this.color;
}
};
//Get the value of object property
console.log(car.type); //Output: Toyota
console.log(car.model); //Output: Highlander
console.log(car.color); //Output: blue
//get the value of object method
//or in simple language call the function which is stored as an object property
console.log( car.getCarDetails() );
//Output: Toyota Highlander blue
Let's create an object in another way using object constructor
function Car (type, model, color){
this.type = type;
this.model = model;
this.color = color;
this.getDetails = function(){
return this.type + " " + this.model + " " + this.color;
};
}
//Create and object. We can give name of the object
objCar = new Car("Toyota","Highlander","Blue");
console.log(objCar.type); //Output: Toyota
console.log(objCar.model); //Output: Highlander
console.log(objCar.color); //Output: blue
console.log( objCar.getDetails() );
//Output: Toyota Highlander blue