JavaScript Loops
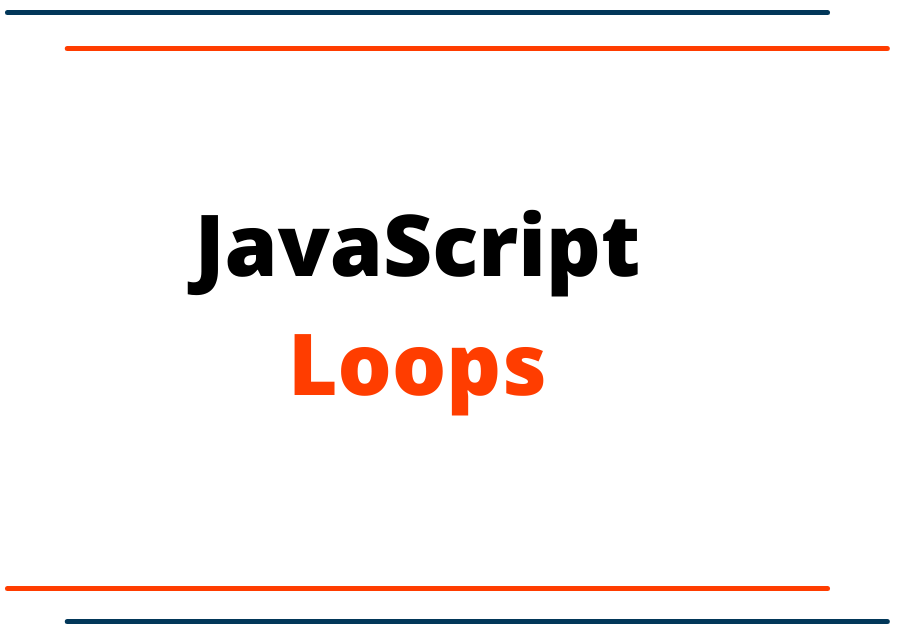
JavaScript Loops
If you have to execute the block of the code number of times, then loops can be used to do that job. Like other programming languages, JavaScript loops are used to iterate the piece of code using different kinds of loops. There are five types of loops in JavaScript.
Let’s look at the code example one by one.
For loop
for (initialization; condition; increment)
{
//code to be executed
}
for (i=1; i<=5; i++)
{
console.log(i);
}
//Output: 1,2,3,4,5
While loop
while (condition)
{
// code to be executed
}
var i=1;
while (i<=5)
{
console.log(i);
i++;
}
//Output: 1,2,3,4,5
Do while loop
do{
//code to be executed
} while (condition);
var i=1;
do {
console.log(i); //At least run it one time
i++;
}while (i<=5);
//Output: 1,2,3,4,5
For In loop
for (key in object) {
// code block to be executed
}
var person = {firstname:"Johna", lastname:"Doea", age:20};
for (var x in person) {
console.log(person[x]);
}
//Output: "Johna","Doea",20
For of loop
const cars = ["Toyota", "Honda", "BMW"];
for (let x of cars) {
console.log(x);
}
//Output: "Toyota", "Honda", "BMW"