JavaScript If Else Conditional Statement
Last Updated on May 06, 2022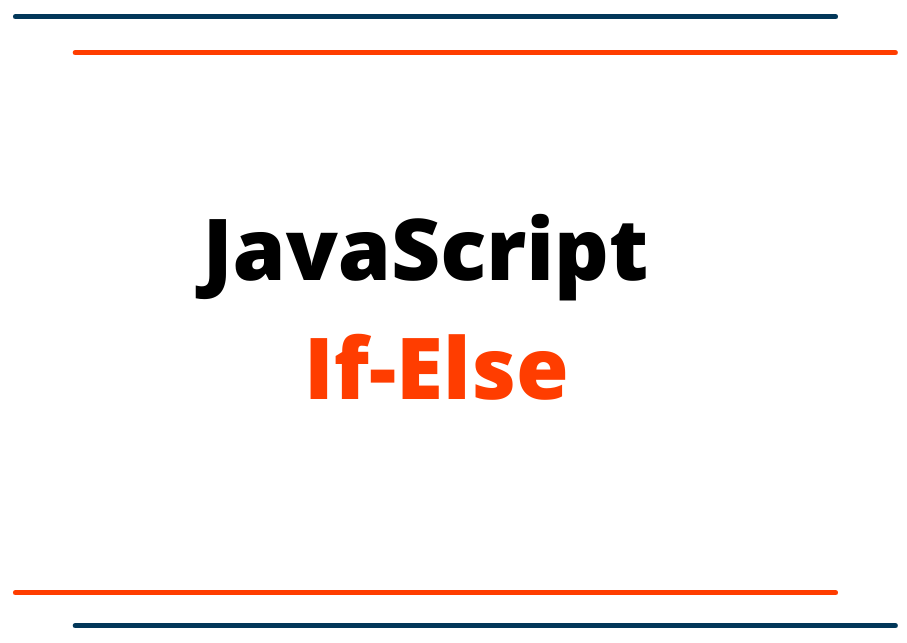
JavaScript If-Else Statement
A conditional statement is useful and most used to check and perform different actions of code based on different conditions. The conditional statement is the very basic building block of any programing language.
Let’s look at an example of how to write the conditional statement.
if (condition)
{
//Your statement goes here;
//This statement will be executed if the condition is true
}
else
{
//Your other statement goes here;
//This statement will be executed if the condition is false
}
//You can also have more than one conditions
if (condition1)
{
//statement one
}
else if(condition2)
{
//statement two
}
else if(condtion3)
{
//statement three
}
else
{
//statement four or default statement if any of the above conditions are false
}
//You can even have two conditions to check using logical operators
if (condition1 && condition2)
{
//statement one if condtion1 and condition2 both are true
}
else if(condition3 || condition4)
{
//statement two if condition3 or condition4 any of one is true
}
else
{
//default statement if none of the above conditions are true
}
let luckyNumber = 5;
if(luckyNumber == 5)
{
console.log("You are lucky!");
}
else
{
console.log("Please try again!");
}
//Output: You are lucky!
let luckyNumber = 6;
if(luckyNumber == 5)
{
console.log("You are lucky!");
}
else
{
console.log("Please try again!");
}
//Output: Please try again!
let luckyNumber = 5;
if(luckyNumber != 5)
{
console.log("You are lucky!");
}
else
{
console.log("Please try again!");
}
//Output: Please try again!
Look at that, you have many ways to check the condition and execute the code based on the condition.