JavaScript Function
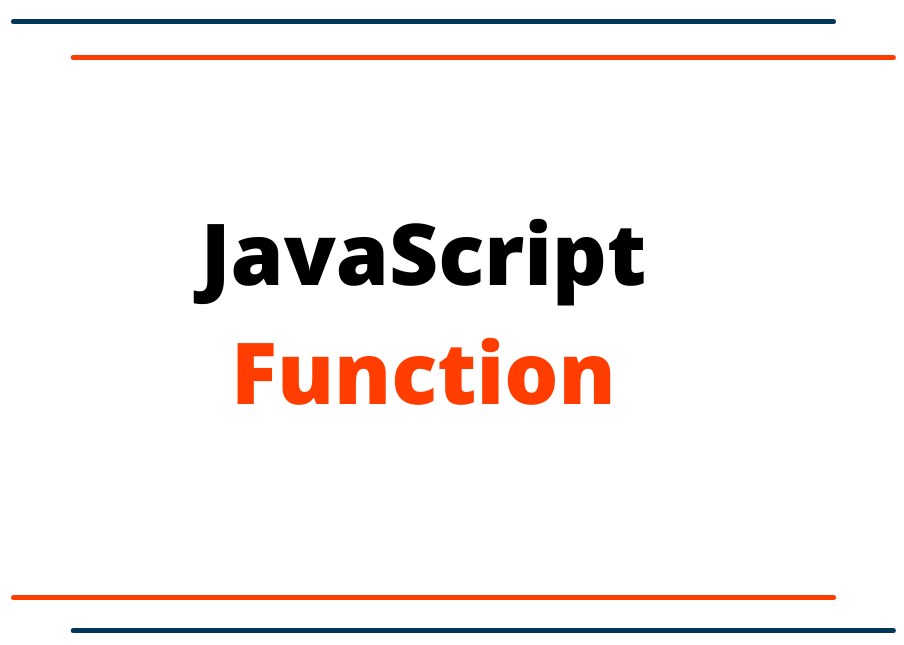
JavaScript Function
The JavaScript function is a programming language construct that allows the programmer to define a group of statements to be executed. The JavaScript function is a block of code to perform a particular task. It can take parameters, execute code when call and return a value.
The function is declared with the keyword function followed by the name of the function, parentheses (which may contain parameters), and curly brackets containing its body.
Let's look at some examples of how to declare the function and call it.
function function_of_the_function(parameter1, parameter2) {
// code to be executed
}
Function without any parameters. Simply it can be define and call it as much as you need. That's the benefit of using function so, we can reuse the same code many times as we need.
function doSomething()
{
return 5 * 5;
}
console.log(doSomething());
//Output: 25
Let's take a look at another example using parameters.
function doSomething(par1, par2)
{
return par1 * par1;
}
console.log(doSomething(5,5));
//Output: 25
You don't have to give the same name of the parameters. You can give any name you want.
function doSomething(x, y)
{
return x * y;
}
console.log(doSomething(5,5));
//Output: 25
You can call the function however you want
function doSomething(x, y)
{
return x * y;
}
let doSomethingVar = doSomething(5,5);
console.log(doSomethingVar);
//Output: 25
function doSomething(x, y)
{
return x * y; //Return the result from here
return x + y; //No way to execute it since it was already returned
}
let doSomethingVar = doSomething(5,5);
console.log(doSomethingVar);
//Output: 25
How to use a local variable.
function doSomething(x, y)
{
let z = 5; //Local Var which can only be use by doSomething function. Other functions cannot use this local variable.
return x + y + z;
}
let doSomethingVar = doSomething(5,5);
console.log(doSomethingVar);
//Output: 15
How to use a global variable.
let z = 5; //Global Var which is available to use by any functions
function doSomething(x, y)
{
return x + y + z;
}
let doSomethingVar = doSomething(5,5);
//Let's create another doSomething2 function and use the same global variable
function doSomething2(x, y)
{
return x + y + z;
}
console.log(doSomethingVar); //Output:15
console.log(doSomething2(2 ,2)); //Output: 9