C# Loops
Last Updated on May 10, 2022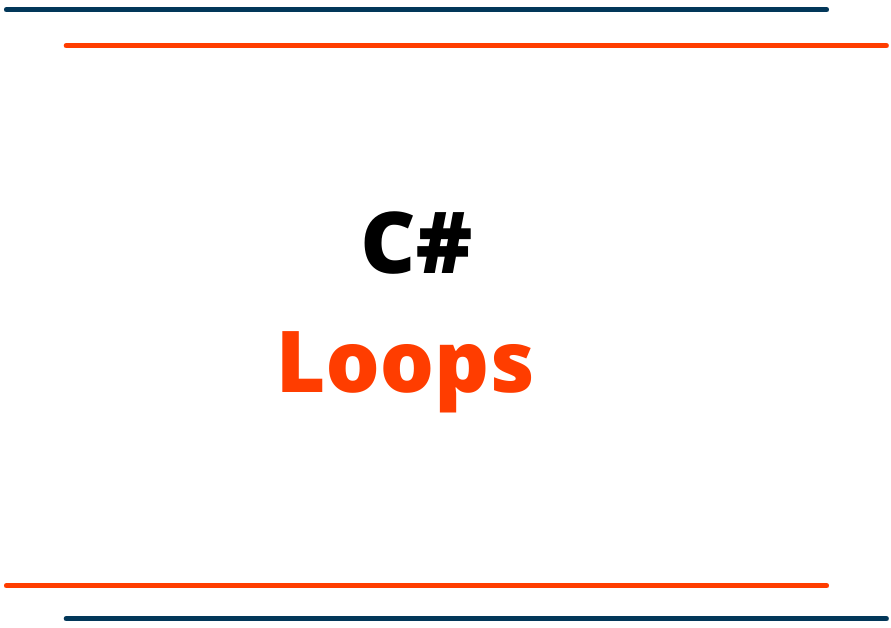
C# Loops
Loops are a type of programming language that are used to repeat tasks. If we need to execute a block of code statement multiple times, then we can use any available loops.
C# For Loop
//For Loop Syntax
for (initialization; condition; increment/decrement)
{
// code block to be executed
}
using System;
public class Program
{
public static void Main()
{
for(int i = 0; i <= 10; i++){
Console.WriteLine(i);
}
}
}
//Output: 0 1 2 3 4 5 6 7 8 9 10
using System;
public class Program
{
public static void Main()
{
for(int i = 0; i <= 20; i = i + 2){
Console.WriteLine(i);
}
}
}
//Output: 0 2 4 6 8 10 12 14 16 18 20
We can also have the nested loops we need while developing the application.
using System;
public class Program
{
public static void Main()
{
for(int i=1; i<=2; i++){
for(int j=1; j<=2; j++){
Console.WriteLine(i+" "+j);
}
}
}
}
//Output:
1 1
1 2
2 1
2 2
The foreach loop in C#
foreach (type variableName in ArrayLists)
{
// block of code to be executed
}
using System;
public class Program
{
public static void Main()
{
string[] listOfProgrammingLang = {"JavaScript", "Python", "Csharp", "AspDotNet", "HTML"};
foreach (string lang in listOfProgrammingLang)
{
Console.WriteLine(lang);
}
}
}
//Output: JavaScript Python Csharp AspDotNet HTML
The while loop in C#
//C# while loop syntax
while (condition)
{
//code to be executed
}
using System;
public class Program
{
public static void Main()
{
int i = 0;
while(i < 6){
Console.WriteLine(i);
i++;
}
}
}
//Output:0 1 2 3 4 5
The do-while loop in C#.
If we want to execute the code at least once then we can use the do-while loop.
//do while loop syntax
do
{
//code to be executed
}
while (condition);
using System;
public class Program
{
public static void Main()
{
int i = 1;
do
{
Console.WriteLine(i);
i++;
}
while (i <= 5);
}
}
//Output: 1 2 3 4 5