C# Variables
Last Updated on May 09, 2022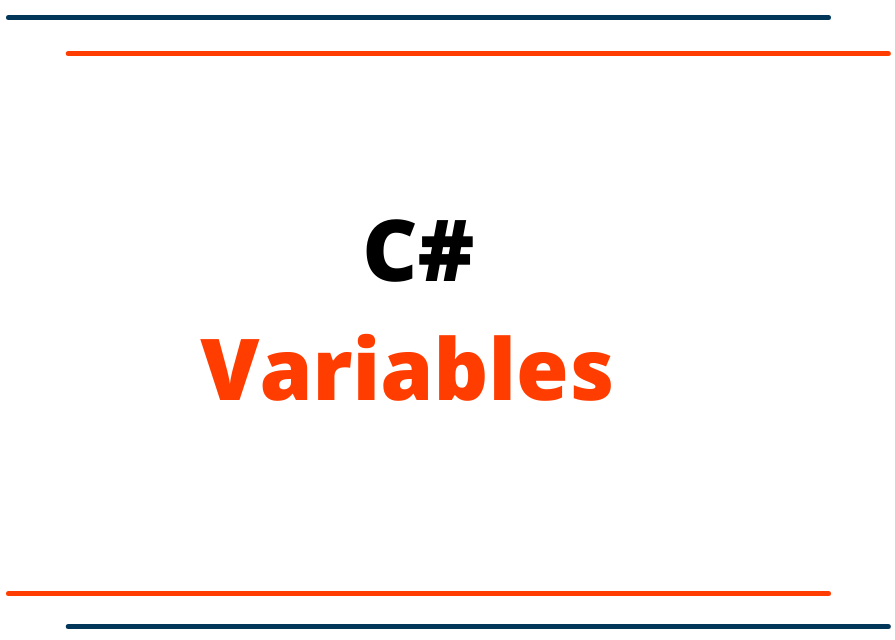
C# Variables
The variable is a container to hold or store the data values. We can use the stored data value as much as we need during the development of the project.
There are different kinds of variable types of available defined with different keywords in C#.
Let's see how to create a variables with an example
//Must have declare the variable with type and name of the variable
type NameOfVariable = value;
int varNum = 10;
double varDoubleNum = 10.55;
string varText = "Learning C-Sharp";
char varLetter = 'W';
bool varBool = true;
//Access or display the variable assigned value
Console.WriteLine(varNum); //Output: 10
Console.WriteLine(varDoubleNum); //Output: 10.55
Console.WriteLine(varText); //Output: Learning C-Sharp
Console.WriteLine(varLetter); //Output: W
Console.WriteLine(varBool); //Output: True
Example of code in Asp.Net Console project.
using System;
public class Program
{
public static void Main()
{
int varNum = 10;
double varDoubleNum = 10.55;
string varText = "Learning C-Sharp";
char varLetter = 'W';
bool varBool = true;
Console.WriteLine(varNum); //Output: 10
Console.WriteLine(varDoubleNum); //Output: 10.55
Console.WriteLine(varText); //Output: Learning C-Sharp
Console.WriteLine(varLetter); //Output: W
Console.WriteLine(varBool); //Output: True
}
}