C# Properties
Last Updated on June 11, 2022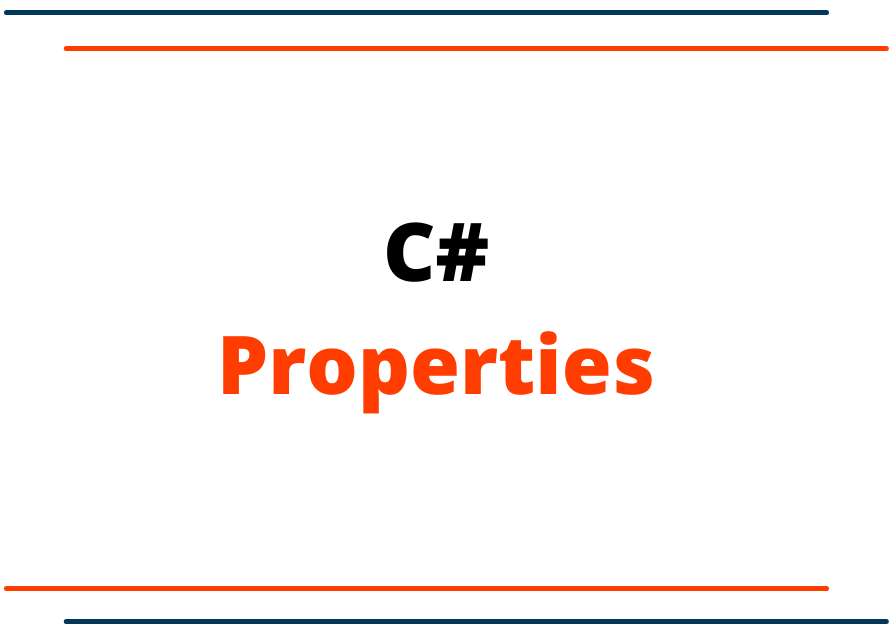
C# Properties
In C# property is the member of the class that provides the flexibility mechanism for classes to expose the class's private members. Also, we need to understand encapsulation is to hide sensitive data from the outside world.
Encapsulation is the one most essential pillar in the OOP world. Object-Oriented Programming languages encapsulation means that any sensitive data need to be private and not accessed and modified by others.
In the following example, we have one private property that has been declared but that is not able to access after creating the object.
using System;
namespace ConsoleAppDemo
{
class Computer {
private string Brand;
}
class Program
{
static void Main(string[] args)
{
Computer computer = new Computer();
//computer.??? //Cannot access the priviate Brand property
Console.ReadKey();
}
}
}
To access the private property, there are some alternate ways. Let's see those with an example.
Example one with getter and setter.
using System;
namespace ConsoleAppDemo
{
class Computer {
private string Brand;
public string BrandGetterSetter
{
get { return Brand; }
set { Brand = value; }
}
}
class Program
{
static void Main(string[] args)
{
Computer computer = new Computer();
computer.BrandGetterSetter = "Lenovo"; //Assign the value to the private property
Console.ReadKey();
}
}
}
There is another shortcut way to produce the same result as the above example but has less code and clean code than the above example.
using System;
namespace ConsoleAppDemo
{
class Computer {
public string Brand { get; set; }
}
class Program
{
static void Main(string[] args)
{
Computer computer = new Computer();
computer.Brand = "Lenovo"; //Assign the value to the private property
Console.ReadKey();
}
}
}
Any method can be applied to access the private fields of the class.