C# OOP Intro
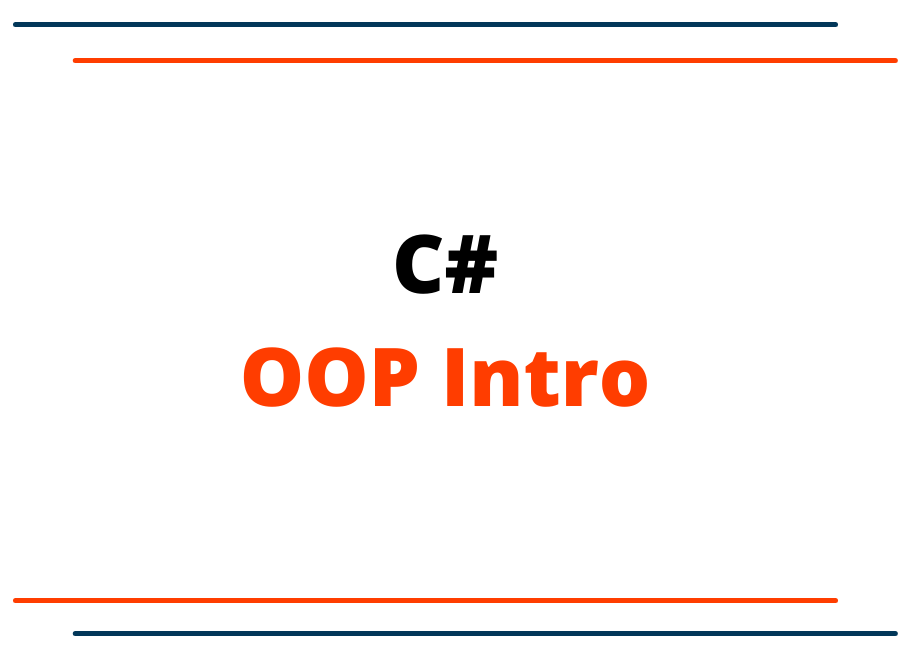
C# OOP Intro
C# is the object-oriented language which means we will build our application based on objects based on the classes. For the beginner, it may little confused about why we need object-oriented programming language and what are the benefits of implementing the OOP in the application.
Don’t be confused because it’s nothing other than the rules for the programmer that can help to build a clean application. As a programmer, we need to build an application that can easily be maintained, code reusability, and scalability that’s the reason we have OOP that can help us to build the application by following some OOP rules.
What is the class?
The class is the blueprint or template of an object. The class has some properties, methods, construction, etc. Based on the class we can create the object. Let’s see the example of class so, it makes more sense.
Let’s create the console application in visual studio and we will write our first class in the program.cs file.
class Computer {
//properties
public int Id;
public string brand;
//Method
public void start() {
Console.WriteLine("Computer can start.");
}
}
Next, let's see how to create an object based on the Computer class which we just have created.
Note: The main entry point of C# programming is the Main() method. First, we have created the class within the same namespace. We will see what is the namespace and the access modifier in later but for now, just try to learn about the class and the object.
using System;
namespace ConsoleAppDemo
{
class Computer {
//properties
public int Id;
public string brand;
//Method
public void start() {
Console.WriteLine("Computer can start.");
}
}
class Program
{
static void Main(string[] args)
{
//Create an object
Computer computer = new Computer();
//Assigned the property value
computer.Id = 1;
computer.brand = "Lenovo";
//Call the method
computer.start();
Console.ReadKey();
}
}
}
Output:
Computer can start.
Let's try another example to print the passing property values.
using System;
namespace ConsoleAppDemo
{
class Computer {
//properties
public int Id;
public string brand;
//Method
public void Intro() {
Console.WriteLine("Computer Id: {0} and the Brand: {1}", Id, brand);
}
}
class Program
{
static void Main(string[] args)
{
//Create an object
Computer computer = new Computer();
//Assigned the property value
computer.Id = 1;
computer.brand = "Lenovo";
//Call the method
computer.Intro();
Console.ReadKey();
}
}
}
Output:
Computer Id: 1 and the Brand: Lenovo