C# Methods
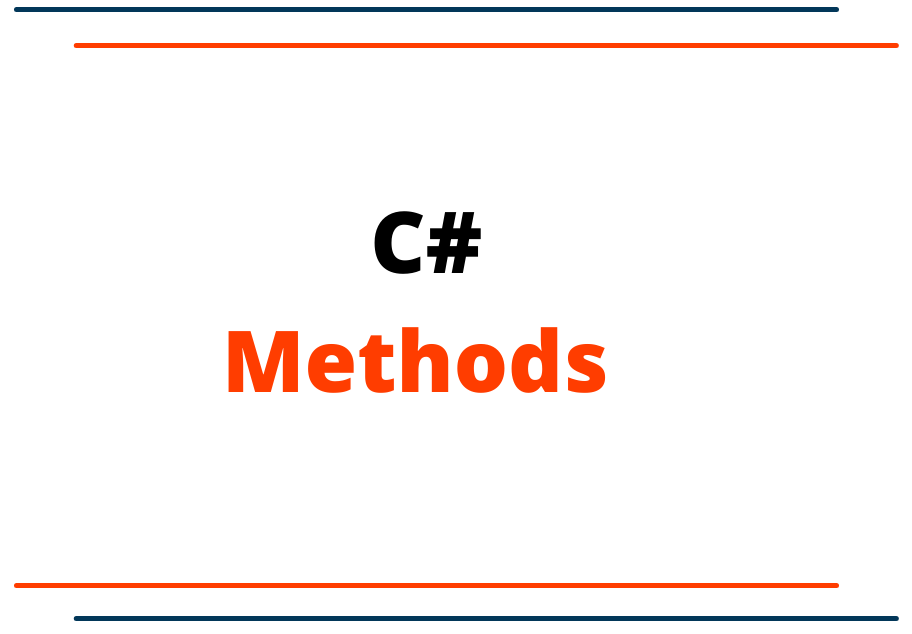
C# Methods
A method is a block of code to define our logic once and use it many times as we need in our application. If we write a set of code one time and re-use it many times to perform certain actions it would be helpful to us to maintain our application and easy for the developer to save their time so, we don't have to re-write the code to perform same tasks again and again.
A method is also known as a function same as like other programming languages. C# is an Object-Oriented Programming language that's why any OOP-based programming language functions are known as methods. Don't be confused, functions and methods are the same.
Let's look at how to create a method/function in C#.
//Method code syntax
accesstype access_method returntype Name_of_the_method(parameter1,parameter2,parameter3,parametesMore)
{
// block of code to execute
// return statement if any
}
C# method without using parameters and and no return type.
using System;
public class Program
{
public static void Main()
{
doWork(); //Calling Static Method
}
//Static Method
public static void doWork()
{
Console.WriteLine("Method without parameters and no return type!");
}
}
//Output: Method without parameters and no return type!
The same example of the method without parameters and no return type but this time let's do without static method type so, we can create an object to call or use the method.
using System;
public class Program
{
public static void Main()
{
Program program = new Program(); //Create an Object
program.doWork(); //Calling the method
}
public void doWork()
{
Console.WriteLine("Method without parameters and no return type!");
}
}
//Output: Method without parameters and no return type!
C# method with using parameters and and no return type.
using System;
public class Program
{
public static void Main()
{
doWork("Method with parameters and no return type!"); //Calling the method with passing the required argument
}
public static void doWork(string msg)
{
Console.WriteLine(msg);
}
}
//Output: Method with parameters and no return type!
The same example of the method with parameters and no return type but this time let's do without static method type so, we can create an object to call or use the method.
using System;
public class Program
{
public static void Main()
{
Program program = new Program(); //Create an Object
program.doWork("Method with parameters and no return type!"); //Calling the method with passing the required argument
}
public void doWork(string msg)
{
Console.WriteLine(msg);
}
}
//Output: Method with parameters and no return type!
This time let's see the example of using parameters and return type. Note: replacing the void with string type because we are returning the string as a message.
using System;
public class Program
{
public static void Main()
{
//Calling the method with passing the required argument
Console.WriteLine( doWork("Method with parameters and string return type!") );
}
public static string doWork(string msg)
{
return msg; //return the msg parameter
}
}
//Output: Method with parameters and string return type!
The same example of the method with parameters and return type but this time let's do without static method type so, we can create an object to call or use the method.
using System;
public class Program
{
public static void Main()
{
Program program = new Program(); //Create an Object
//Calling the method with passing the required argument
Console.WriteLine( program.doWork("Method with parameters and string return type!") );
}
public string doWork(string msg)
{
return msg;
}
}
//Output: Method with parameters and string return type!