C# Indexer
Last Updated on June 11, 2022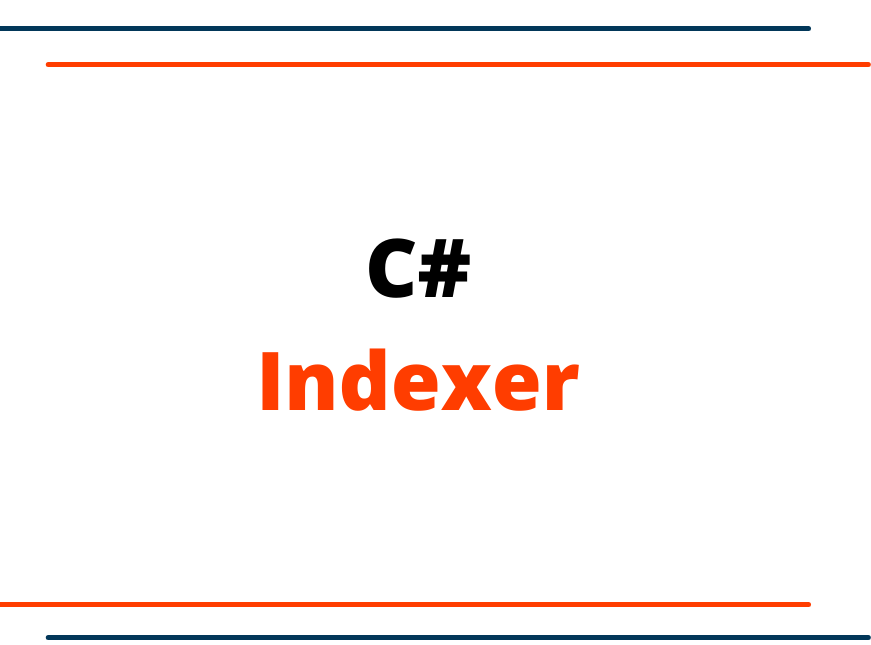
C# Indexers
In C# an Indexer is a special type of property that allows a class or structure to be accessed the same way as an array for its internal collection. We can get or set the index value without explicitly specifying a type or instance member.
To define the indexer, we need to declare an array to store the data elements and we need to also define the indexer to allow the client code to use the square brackets [] notation.
Let’s look at an example of Indexer in C#.
using System;
namespace ConsoleAppDemo
{
class DemoIndexer {
private int[] arrayCollection = new int[5];
public int this [int indexer] {
get {
return arrayCollection[indexer];
}
set {
arrayCollection[indexer] = value;
}
}
}
class Program
{
static void Main(string[] args)
{
DemoIndexer demoIndexer = new DemoIndexer();
demoIndexer[0] = 5;
demoIndexer[1] = 10;
demoIndexer[2] = 15;
for (int i = 0; i < 3; i++)
{
Console.WriteLine(demoIndexer[i]);
}
Console.ReadKey();
}
}
}
Output:
5 10 15