C# If Else
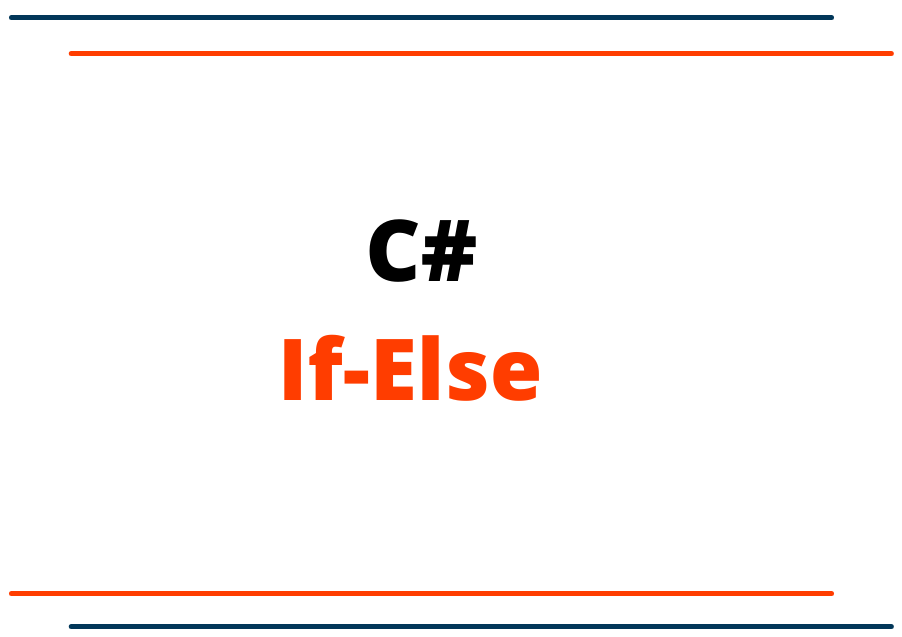
C# If-Else
The if statement is to test the given condition and execute the specific code based on the condition whether it's true or false. Same as any other programming language, C# also provides various types of if statements.
Let's take a look at an example of an if statement first and then we will see the If-Else statement code.
//Code syntax
if (condition)
{
// If the condition is true then execute the block of code
}
}
using System;
public class Program
{
public static void Main()
{
//Checking 10 is greater than 5 or not?
if (10 > 5 )
{
Console.WriteLine("Yes, the given condition is true");
}
//Output: Yes, the given condition is true
}
}
using System;
public class Program
{
public static void Main()
{
//Using variables
int x = 10;
int y = 5;
//Checking 10 is greater than 5 or not?
if (x > y )
{
Console.WriteLine("Yes, the given condition is true");
}
//Output: Yes, the given condition is true
}
}
Let's use the If-Else statement.
//Code syntax using else
if (condition)
{
// If the condition is true then execute the block of code
}
else
{
// If the condition is false then execute the block of code
}
}
using System;
public class Program
{
public static void Main()
{
//Using variables
int x = 10;
int y = 50;
//Checking 10 is greater than 50 or not?
if (x > y )
{
Console.WriteLine("Yes, the given condition is true");
}
else
{
Console.WriteLine("No, the given condition is false");
}
//Output: No, the given condition is false
}
}
We can also have a nested type of If statement. Let's take an example of it.
using System;
public class Program
{
public static void Main()
{
//Using variables
int x = 10;
int y = 5;
//Checking 10 is greater than 5 or not?
if (x > y )
{
//Nested If-Else
if(x == 10)
{
Console.WriteLine("Yes, the given condition is true and the value of x is 10");
}
else
{
Console.WriteLine("Yes, the given condition is true but the value of x is not 10");
}
}
else
{
Console.WriteLine("Yes, the given condition is false");
}
//Output: Yes, the given condition is true and the value of x is 10
}
}
In C#, we can also have a ladder-type of the If-Else-If statement.
using System;
public class Program
{
public static void Main()
{
//Using variables
int nNum = 35;
if(nNum >= 0 && nNum < 25)
{
Console.WriteLine("nNum value is between 0 - 25");
}
else if(nNum >= 25 && nNum < 50)
{
Console.WriteLine("nNum value is between 25 - 50");
}
else if(nNum >= 50 && nNum < 75)
{
Console.WriteLine("nNum value is between 50 - 75");
}
else if(nNum > 75 && nNum < 100)
{
Console.WriteLine("nNum value is between 75 - 100");
}
else
{
Console.WriteLine("nNum value is greater than 100");
}
//Output: nNum value is between 25 - 50
}
}