C# Generics
Last Updated on June 13, 2022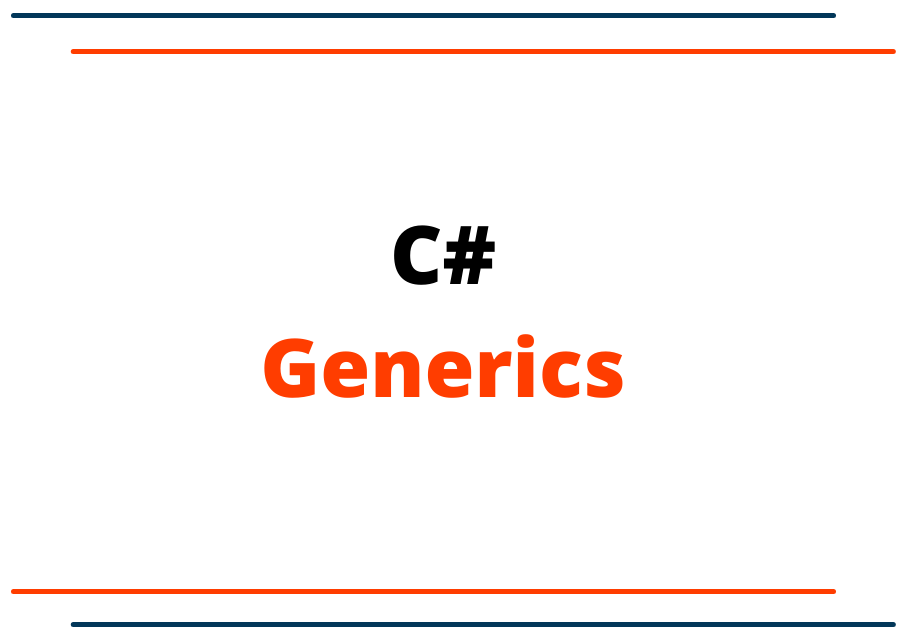
C# Generics
In C# generics means not specific to a particular data type. We can define type-safe data structures without the specific data type. It helps significant performance boost and higher quality code because we get to reuse data processing algorithms without duplication of type-specific code.
The generic class can be defined with angle <> brackets. In C# we can define generic classes, interfaces, abstract classes, fields, methods, static methods, properties, events, delegates, etc.
Let’s see an example with single parameter in generic class.
using System;
namespace ConsoleAppDemo
{
class DataProcess {
public T Data { get; set; }
}
class Program
{
static void Main(string[] args)
{
//Assign value with string data type
DataProcess dataProcess = new DataProcess();
dataProcess.Data = "Data is processing";
//Assign value with int data type
DataProcess dataProcess1 = new DataProcess();
dataProcess1.Data = 100;
Console.ReadKey();
}
}
}
Let's see another example with multiple parameters in generic class.
using System;
namespace ConsoleAppDemo
{
class DataProcess<TKey, TValue> {
public TKey DataKey { get; set; }
public TValue DataValue { get; set; }
}
class Program
{
static void Main(string[] args)
{
DataProcess<int, string> dataProcess = new DataProcess<int, string>();
dataProcess.DataKey = 1;
dataProcess.DataValue = "Software Engineer";
DataProcess<string, string> dataProcess1 = new DataProcess<string, string>();
dataProcess1.DataKey = "SE";
dataProcess1.DataValue = "Software Engineer";
Console.ReadKey();
}
}
}
In the above example, we see how to define the generic class but we can also define generic interfaces, abstract classes, fields, methods, static methods, properties, events, delegates, etc.