C# Arrays
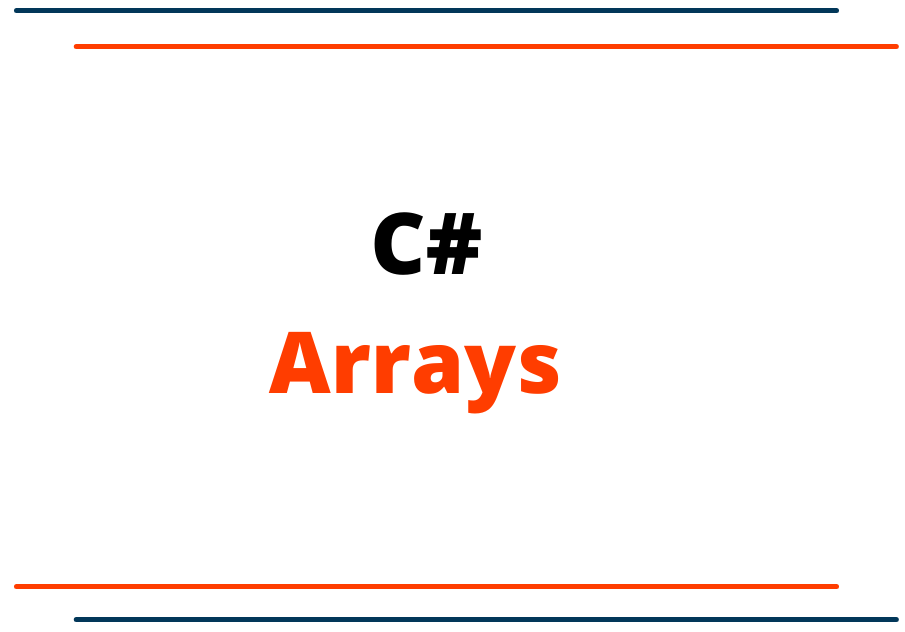
C# Arrays
The Arrays are used to store similar types of multiple values same as in other programming languages. We can hold the data using variables, but the variable that is special can hold a single value at a time whereas the array can hold multiple values.
There are three types of the array in C# which are listed below.
- Single Dimensional Array
- Multidimensional Array
- Jagged Array
Single Dimensional Array Declaration
There are several ways to declare the array in C# programming languages. Let’s look at it with an example.
//Array declaration syntax in C#
string[] strArray; // string type
int[] numArray; // integer type
Declaration with the new keyword and with a fixed size of the array.
string[] listOfProgrammingLang = new string[5]{ "JavaScript", "Python", "Csharp", "AspDotNet","HTML" };
Declaration with the new keyword and with using the var keyword.
var listOfProgrammingLang = new string[]{ "JavaScript", "Python", "Csharp", "AspDotNet","HTML" };
Declaration without new keyword and without using the var keyword. Declare with the type of array and assign the values. This is the best shortcut way to declare the array in the C# programming language.
string[] listOfProgrammingLang = { "JavaScript", "Python", "Csharp", "AspDotNet","HTML" };
Once we know how to create an array, we also need to how to access the element of an array. Let's see some examples how we can access the value of an array. Note array index always start from number 0.
Using Index to access the array elements.
public class Program
{
public static void Main()
{
string[] listOfProgrammingLang = { "JavaScript", "Python", "Csharp", "AspDotNet","HTML" };
Console.WriteLine(listOfProgrammingLang[0]); //Output: JavaScript
Console.WriteLine(listOfProgrammingLang[1]); //Output: Python
Console.WriteLine(listOfProgrammingLang[2]); //Output: Csharp
Console.WriteLine(listOfProgrammingLang[3]); //Output: AspDotNet
Console.WriteLine(listOfProgrammingLang[4]); //Output: HTML
}
}
Using for loop to access the array elements.
using System;
public class Program
{
public static void Main()
{
string[] listOfProgrammingLang = { "JavaScript", "Python", "Csharp", "AspDotNet","HTML" };
for (int i = 0; i < listOfProgrammingLang.Length; i++)
{
Console.WriteLine(listOfProgrammingLang[i]);
}
}
}
//Output: JavaScript Python Csharp AspDotNet HTML
Using foreach loop to access the array elements.
using System;
public class Program
{
public static void Main()
{
string[] listOfProgrammingLang = { "JavaScript", "Python", "Csharp", "AspDotNet","HTML" };
foreach(var item in listOfProgrammingLang)
{
Console.WriteLine(item);
}
}
}
//Output: JavaScript Python Csharp AspDotNet HTML
Multidimensional Arrays
In C# Multidimensional Arrays are the matrix type of array which store data in tabular form like row and column. Multidimensional Arrays can support up to 32 dimensions in C#. Let's take a look at some of the examples how to create the Multidimensional Arrays in C#,
int[,] a2d; // 2D array
int[, ,] a3d; // 3D array
int[, , ,] a4d ; // 4D array
//We can go up to 32dD........
There are 3 ways to initialize the multidimensional array in C#
Declare the multidimensional array with new keyword and with fixed size.
int[,] aNums = new int[2,2]= { { 1, 2 }, { 3, 4 } };
Declare the multidimensional array with new keyword but without giving the fixed size.
int[,] aNums = new int[,]{ { 1, 2 }, { 3, 4 } };
Declare the multidimensional array without a new keyword and the fixed size. This is also one of the best and shortcut ways to declare the multidimensional array in C#.
int[,] aNums = { { 1, 2 }, { 3, 4 } };
Once we know how to create the array then we should also know how to access the array values.
using System;
public class Program
{
public static void Main()
{
int[,] aNums = { { 1, 2 }, { 3, 4 } };
for(int i=0;i<2;i++){
for(int j=0;j<2;j++){
Console.Write(aNums[i,j]+" ");
}
Console.WriteLine(" "); //Line break
}
}
}
Output:
1 2 3 4
Jagged Arrays
C# language also supports Jagged Arrays which is also known as the "array of arrays". The jagged array can store the array as an element. Let's see how to declare the Jagged Array.
//Declare jagged array
int[][] arr = new int[2][];
//Initialize the jagged array
arr[0] = new int[2];
arr[1] = new int[4];
Let's see another example of Jagged Array and get the elements of it.
using System;
public class Program
{
public static void Main()
{
// Declare the array
int[][] aNums = new int[2][];
//Assign the arrays in above aNums Jagged Array
aNums[0] = new int[3]{1, 2, 3};
aNums[1] = new int[4]{4, 5, 6, 7 };
//Get the elements of an array by using index
Console.WriteLine(aNums[0][0]);
Console.WriteLine(aNums[0][1]);
Console.WriteLine(aNums[0][2]);
Console.WriteLine("----------");
Console.WriteLine(aNums[1][0]);
Console.WriteLine(aNums[1][1]);
Console.WriteLine(aNums[1][2]);
Console.WriteLine(aNums[1][3]);
}
}
Output:
1 2 3 ---------- 4 5 6 7
Another example of retrieving the array elements using a loop
using System;
public class Program
{
public static void Main()
{
// Declare the array
int[][] aNums = new int[2][];
//Assign the arrays in above aNums Jagged Array
aNums[0] = new int[3]{1, 2, 3};
aNums[1] = new int[4]{4, 5, 6, 7 };
for (int i = 0; i < aNums.Length; i++)
{
for (int j = 0; j < aNums[i].Length; j++)
{
System.Console.Write(aNums[i][j]+" ");
}
System.Console.WriteLine();
}
}
}
Output:
1 2 3 4 5 6 7