Angular ngIf
Last Updated on May 20, 2022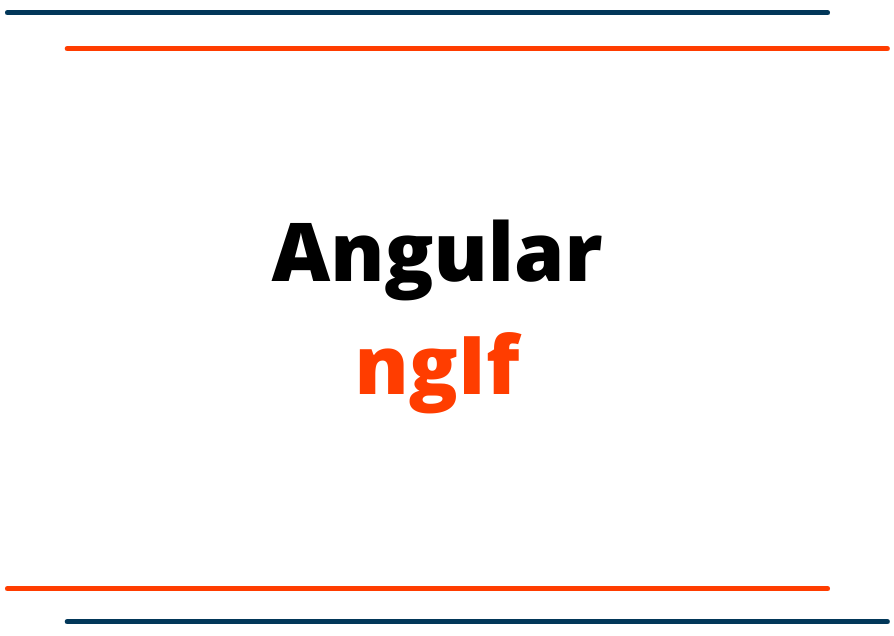
Angular ngIf
In Angular ngIf structural directive allows us to add or remove the HTML element based on some conditions. Just like other programming languages, it provides all kinds of If-Else statements.
Let’s look at code syntax and some code examples of using ngIf.
Hide or display based on the condition is true/false
Let's take a look at the code example.
import { Component } from '@angular/core';
@Component({
selector: 'app-demoComponent',
template: `
Conditionally display or hide the paragraph.
`
})
export class DemoComponent {
bCondition = true; //Could be true or false
constructor(){
//Constructor
}
}
Based on the bConditon value, the paragraph will be hidden or shown.
Another example with ngIf else.
import { Component } from '@angular/core';
@Component({
selector: 'app-demoComponent',
template: `
Dispalay if bCondtion value is true.
Dispalay if bCondtion value is false.
`
})
export class DemoComponent {
bCondition = false; //Could be true or false
constructor(){
//Constructor
}
}
In the above code example, the paragraph will be displayed or hidden based on the bCondtion value whether true or false. We always put other kinds of the code block for else statement using <ng-template #ReferenceId>code statement........</ng-template>
Another example with ngIf then else.
import { Component } from '@angular/core';
@Component({
selector: 'app-demoComponent',
template: `
Demo of if then else
Dispalay if bCondtion value is true.
Dispalay if bCondtion value is false.
`
})
export class DemoComponent {
bCondition = false; //Could be true or false
constructor(){
//Constructor
}
}
In the above example, the paragraph will be displayed or hidden based on the condition given. If the condition is true then the then-block template paragraph will be displayed otherwise else-block template paragraph will be displayed.