Angular ngFor
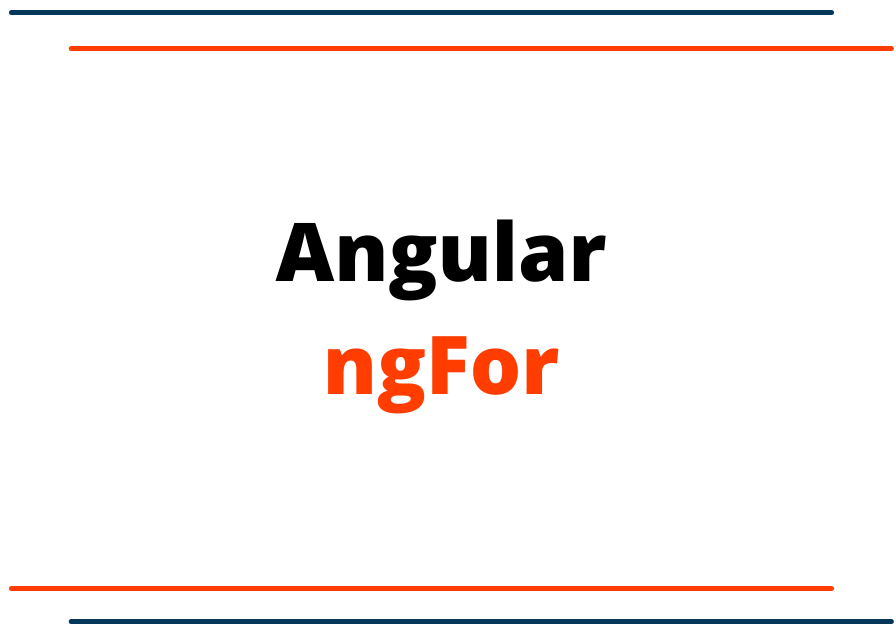
Angular ngFor
In Angular ngFor structural directive allows us to iterate over the collection of an array, list, etc. ngFor directive helps us to create the HTML elements like table rows or some kinds of list of data values from the array. The ngFor works are like the For-Loop statement in other programming languages.
Let’s look at code syntax and some code examples of using ngFor.
import { Component } from '@angular/core';
@Component({
selector: 'app-demoComponent',
template: `
- {{lang}}
`
})
export class DemoComponent {
//List of some programming languages as an array
listOfProgrammingLang = ["JavaScript", "Python", "Csharp", "AspDotNet", "HTML"];
constructor(){
//Constructor
}
}
As an output, we will see the list of array values.
JavaScript Python Csharp AspDotNet HTML
Let's see another example of how to iterate the JSON data using ngFor.
import { Component } from '@angular/core';
@Component({
selector: 'app-demoComponent',
template: `
#
Brand
Country
Product Type
{{i+1}} //So it can start from 1 rather than 0
{{computer.Brand}}
{{computer.Country}}
{{computer.Product_Type}}
`
})
export class DemoComponent {
//List of some computer info in Json
listOfComputers = [
{Brand: "Lenovo", Country:"China", Product_Type:"Yoga"},
{Brand: "HP", Country:"United States", Product_Type:"Elitebook"},
{Brand: "Dell", Country:"United States", Product_Type:"Vostro"},
{Brand: "Apple", Country:"United States", Product_Type:"MacBook"},
{Brand: "Asus", Country:"Taiwan", Product_Type:"ZenBook"},
{Brand: "Lenovo", Country:"China", Product_Type:"Legion"}
];
constructor(){
//Constructor
}
}
As an output, we will see the list of all computer information in HTML table format.