Angular Two Way Data Binding
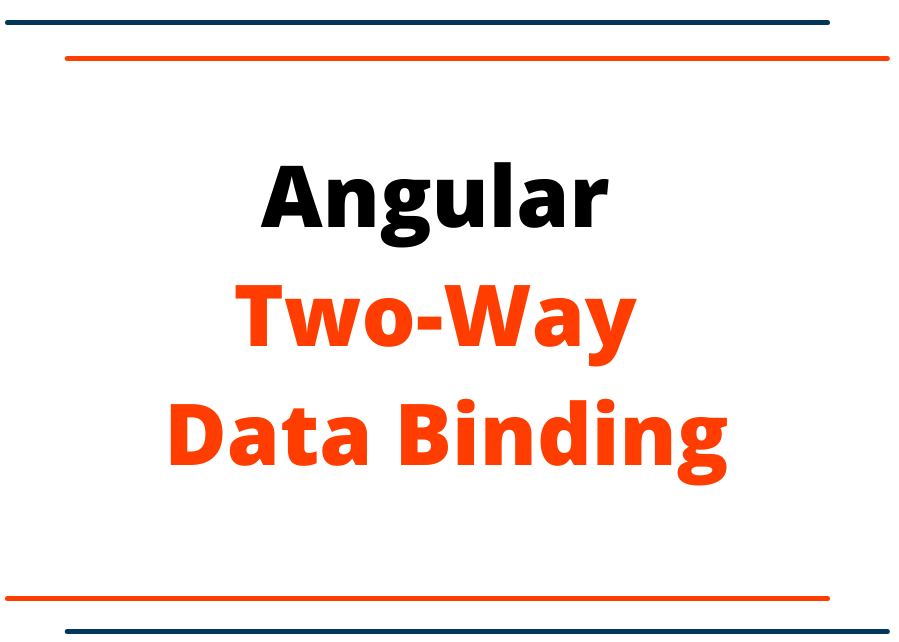
Angular Two-Way Data Binding
Here, we will learn about another data binding technique which is called two-way data binding. Two-Way data binding refers to the sync of the data between view and component. It means if we change the data in one place it’s automatically reflected in another place. Two-Data data binding is the great feather that Angular provides.
Two-Way data binding has special code syntax which is also known as a banana in the box [()]
We need to add the ngModel directive to achieve the two-way data binding which is not part of the Angular core library. To add the ngModel directive, please add the below-highlighted code in the app.model.ts file.
import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { FormsModule } from '@angular/forms'; import { AppRoutingModule } from './app-routing.module'; import { AppComponent } from './app.component'; import { DemoComponent } from './demoComponent/demo.component'; @NgModule({ declarations: [ AppComponent, DemoComponent ], imports: [ BrowserModule, AppRoutingModule, FormsModule ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
After we added the above highlighted code the the app.model.ts file, we are ready to see an example of two-way data binding.
import { Component } from '@angular/core';
@Component({
selector: 'app-demoComponent',
template: `
{{ ramdomText }}
`
})
export class DemoComponent {
ramdomText: any = "Type something";
constructor(){
//Constructor
}
}
As an output, you will see the input field with the text value "Type something" and also the output of it with the same text value. If you type anything in that input field, that will immediately reflect the output text as well.