Angular Services
Last Updated on May 23, 2022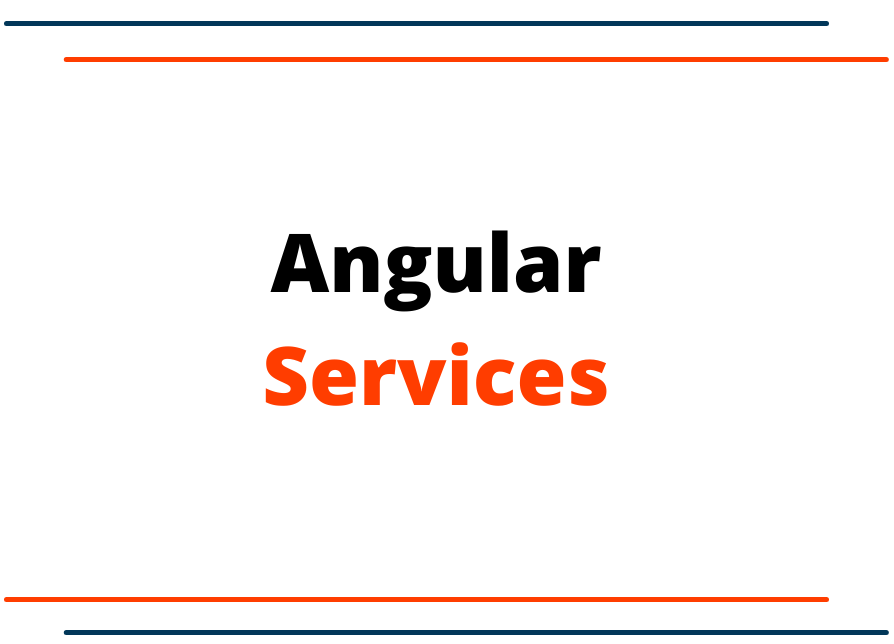
Angular Services
The Services is a piece of reusable code that centralize some kinds of data source or business logic to reuse it across the components or directives. Angular services provide some essential benefits like a force to follow DRY (Don’t Repeat Yourself) principles and the Single Responsibilities Principle.
There are other specific proposals to create and use Angular services.
- The data can be shared across any components or directives.
- Implement application business logic.
- External Interaction like connecting to the database.
- Easy to maintain the application because it is forced to follow DRY principles so, the code will be in one place.
Let's see the real code example about Angular Services.
Suppose, you have a list of products that need to be displayed with more than one component. So, in this case, we can create the services and reuse that services for those components where we would like to display the products.
So, let's create the new file under src/app and name it something like product.service.ts.
export class ProductService {
constructor(){ }
getProducts(){
return [
{Brand: "Lenovo", Country:"China", Product_Type:"Yoga"},
{Brand: "HP", Country:"United States", Product_Type:"Elitebook"},
{Brand: "Dell", Country:"United States", Product_Type:"Vostro"},
{Brand: "Apple", Country:"United States", Product_Type:"MacBook"},
{Brand: "Asus", Country:"Taiwan", Product_Type:"ZenBook"},
{Brand: "Lenovo", Country:"China", Product_Type:"Legion"}
];
}
}
After that, it's time to import that new services to app.modules.ts file so, it can be available global level. New changes are highlighed in below code block.
import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { FormsModule } from '@angular/forms'; import { AppRoutingModule } from './app-routing.module'; import { AppComponent } from './app.component'; import { DemoComponent } from './demoComponent/demo.component'; //Services import {ProductService} from './product.service'; @NgModule({ declarations: [ AppComponent, DemoComponent ], imports: [ BrowserModule, AppRoutingModule, FormsModule ], providers: [ProductService], bootstrap: [AppComponent] }) export class AppModule { }
After importing the service, let's call the method which is returning the hardcoded data set from the product.service.ts file. But in a real application, we will retrieve the data from the database using REST API. For the demo proposal, we have hardcoded the data.
import { Component } from '@angular/core';
import { ProductService } from '../product.service';
@Component({
selector: 'app-demoComponent',
template: `
#
Brand
Country
Product Type
{{i+1}}
{{product.Brand}}
{{product.Country}}
{{product.Product_Type}}
`
})
export class DemoComponent{
products:any = []; //Property that can hold the return data from product.service.ts
//Assigned the method return values which is available in product.service.ts to the local property of this component
//So, that data set can be use in HTML template to display the list of product
constructor(private _productService: ProductService){
this.products = this._productService.getProducts();
}
}
After doing all the above steps, we can run the project to see the result. As an output, we will see the list of products in HTML table format.