Angular Router
Last Updated on May 26, 2022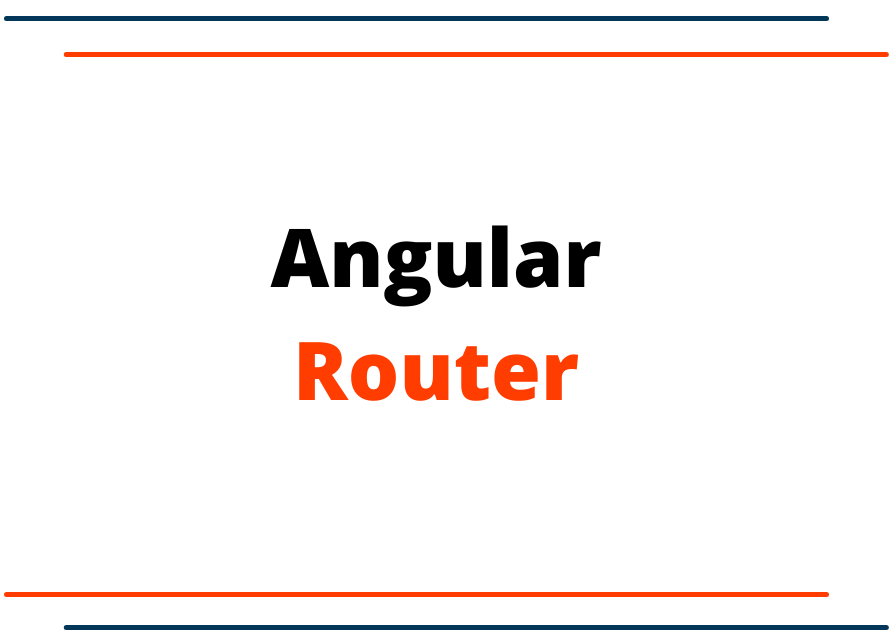
Angular Router
In general language, routing means navigating from one part to another. In Angular router feature allow us to navigate one part of the view to another part of the view. As we know on any website when we click on any of the link buttons, we will be routed from one page to another page. The same as that in Angular we can do similar navigation from one view to another.
The new version of the Angular routing feature will be added while creating the project but in-case if we don’t have it then we can enable it manually. To enable the route in the existing project, we must configure the following thing in our application.
- In the Index.html file, we must add the <base href="/"> in the head section. This code will construct the URL while we are navigating.
AngularApp - Create a new file under the src/app folder and name it app-routing.module.ts and add the following code to configure the Angular routing feature.
//app-routing.module.ts import { NgModule } from '@angular/core'; import { RouterModule, Routes } from '@angular/router'; const routes: Routes = []; @NgModule({ imports: [RouterModule.forRoot(routes)], exports: [RouterModule] }) export class AppRoutingModule { } - Finally, we can import the AppRoutingModule to the app.module.ts file to enable the routing feature for our application.
//app.module.ts import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { FormsModule } from '@angular/forms'; import { HttpClientModule } from '@angular/common/http'; import { AppRoutingModule } from './app-routing.module'; import { AppComponent } from './app.component'; @NgModule({ declarations: [ AppComponent, ], imports: [ BrowserModule, AppRoutingModule, FormsModule, HttpClientModule ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
After completing all the above routing feature enable steps then it's time to see how it works.
Let's create two components for the demo so, we can render the component via URL path. As a demo we have added the inventory.component.ts file under src/app/inventoryComponent folder and also added the department.component.ts file under the src/app/departmentComponent folder.
//inventory.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-inventoryComponent',
template: `
Hello from the Inventory Component
`
})
export class InventoryComponent{
constructor(){ }
}
//department.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-departmentComponent',
template: `
Hello from the Department Component
`
})
export class DepartmentComponent{
constructor(){ }
}
Once, these above steps are completed. Let's add the following code to the app-routing.module.ts file.
//app-routing.module.ts
import { NgModule } from '@angular/core';
import { RouterModule, Routes } from '@angular/router';
import { DepartmentComponent } from './departmentComponent/department.component';
import { InventoryComponent } from './inventoryComponent/inventory.component';
const routes: Routes = [
{path : "department", component: DepartmentComponent},
{path : "inventory", component: InventoryComponent}
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
//Bundle up all the rounting within an Array so, app.module.ts can use it
export const rountingComponents = [
DepartmentComponent,
InventoryComponent
];
In the above code, we have configured the path of the routing and which component to render on that path. All the down of the code, we have exported the array of routing so, we can use it to the app.module.ts file that' is why we don't have to re-import the components file with duplicate code.
//app.module.ts import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { FormsModule } from '@angular/forms'; import { HttpClientModule } from '@angular/common/http'; import { AppRoutingModule, rountingComponents } from './app-routing.module'; import { AppComponent } from './app.component'; import { DemoComponent } from './demoComponent/demo.component'; @NgModule({ declarations: [ AppComponent, rountingComponents ], imports: [ BrowserModule, AppRoutingModule, FormsModule, HttpClientModule ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
After taking all of the above steps. It's time to test the output. Start the application and navigate to each of the components via URL like the following link.
http://localhost:4200/department
http://localhost:4200/inventory
If you didn't see any component view as an output. Make sure you have added <router-outlet></router-outlet> the
Also, we can add some kinds of navigation link so, we can click on that button to navigate to the component view. Just add the following code to the app.component.html file.
We will see the nice and simple navigation link in the browser.