Angular HTTP
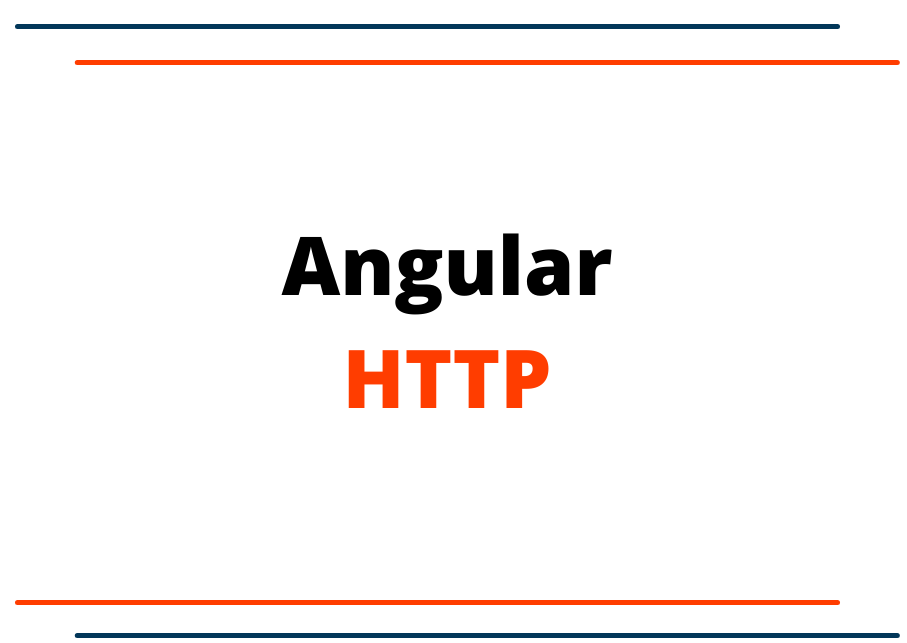
Angular HTTP
We don’t always use the hardcoded data while developing the application. In a real-world application, we may have to communicate with the back-end services to get data, post data, update data, and delete data or full CRUD functionality from the database. So, that’s the reason we are going to learn about the Angular HTTP service.
Angular provides the HTTP Client for Angular applications with some essential features to communicate with REST API services. The HTTP client was introduced after the 4.3 version of Angular and it is available in the package @angular/common/http.
First, we need to understand the mechanism of HTTP requests. Anytime we need to work with API or any kind of data source for our application, in these cases, we can use the Angular Http client services. The response from HTTP Client is observable which means we must subscribe to utilize the data source.
Let’s look at an example. First, we have to import the HttpClientModule to the root module just like the highlighted code.
import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { FormsModule } from '@angular/forms'; import { HttpClientModule } from '@angular/common/http'; import { AppRoutingModule } from './app-routing.module'; import { AppComponent } from './app.component'; import { DemoComponent } from './demoComponent/demo.component'; //Services import {ProductService} from './product.service'; @NgModule({ declarations: [ AppComponent, DemoComponent ], imports: [ BrowserModule, AppRoutingModule, FormsModule, HttpClientModule ], providers: [ProductService], bootstrap: [AppComponent] }) export class AppModule { }
Once we have configured the HttpClientModule to the root module then let's add some more configuration to the service file. For this demo, we have created the product.service.ts.
import { HttpClient } from "@angular/common/http";
import { Injectable } from "@angular/core";
import { Observable } from "rxjs";
@Injectable()
export class ProductService {
private _url:string = "/assets/data/datafile.json";
constructor(private http: HttpClient){ }
getProducts(): Observable<[]>{
return this.http.get<[]>(this._url);
}
}
Notice: We have created the datafile.json instead of using the REST API for this demo. But Let's assume that the file is a kinda REST API which is providing the data source to our application.
datafile.json
[
{"Brand": "Lenovo", "Country":"China", "Product_Type":"Yoga"},
{"Brand": "HP", "Country":"United States", "Product_Type":"Elitebook"},
{"Brand": "Dell", "Country":"United States", "Product_Type":"Vostro"},
{"Brand": "Apple", "Country":"United States", "Product_Type":"MacBook"},
{"Brand": "Asus", "Country":"Taiwan", "Product_Type":"ZenBook"},
{"Brand": "Lenovo", "Country":"China", "Product_Type":"Legion"}
]
Up to now, we have configured the HttpClientModule to the root module and then created the datafile.json where we have stored some data in JSON format. In the product.service.ts file, we are calling that JSON file data file and casting the JSON data to an array, and returning back in the getProducts method. Now it's time to consume the data to the component which is coming from the product.service.ts service file.
import { Component } from '@angular/core';
import { ProductService } from '../product.service';
@Component({
selector: 'app-demoComponent',
template: `
#
Brand
Country
Product Type
{{i+1}}
{{product.Brand}}
{{product.Country}}
{{product.Product_Type}}
`
})
export class DemoComponent{
products:any;
constructor(private _productService: ProductService){
this._productService.getProducts()
.subscribe(data =>
this.products = data
);
}
}
As you notice, in the component file we have Subscribed the Observables and assigned the data to the local variable so, we can have full control of that data. Once we have completed all of the above steps. It's time to see the result. We will still see the list of products in HTML table format that we have been using the HTML template in the component file.
There is another way to re-cast the data in product.service.ts file. Look at of highlighted code.
import { HttpClient } from "@angular/common/http"; import { Injectable } from "@angular/core"; import { Observable } from "rxjs"; @Injectable() export class ProductService { private _url:string = "/assets/data/datafile.json"; constructor(private http: HttpClient){ } getProducts(): Observable<Product[]>{ return this.http.get<Product[]>(this._url); } } //We can create the interface in a different file too and import it into this service file. //For the demo we have created an interface to re-cast the data within this component file. export interface Product{ Brand: string, Country: string, Product_Type: string }
We still see the same results in the browser.
Let's take a look at another example using REST API. For to do that we only have to change some code in the service file and the component file.
//product.service.ts file
import { HttpClient } from "@angular/common/http";
import { Injectable } from "@angular/core";
import { Observable } from "rxjs";
@Injectable()
export class ProductService {
//Using Json Place Holder with limit 10
private _url:string = "https://jsonplaceholder.typicode.com/todos?_limit=10";
constructor(private http: HttpClient){ }
getProducts(): Observable<[]>{
return this.http.get<[]>(this._url);
}
}
//Let's assume todos instead of product
import { Component } from '@angular/core';
import { ProductService } from '../product.service';
@Component({
selector: 'app-demoComponent',
template: `
ID
Title
Completed Type
{{todo.id}}
{{todo.title}}
{{todo.completed}}
`
})
export class DemoComponent{
todos:any;
constructor(private _productService: ProductService){
this._productService.getProducts()
.subscribe(data =>
this.todos = data
);
}
}
We will see the list of todos data in HTML table format.