Angular Event Binding
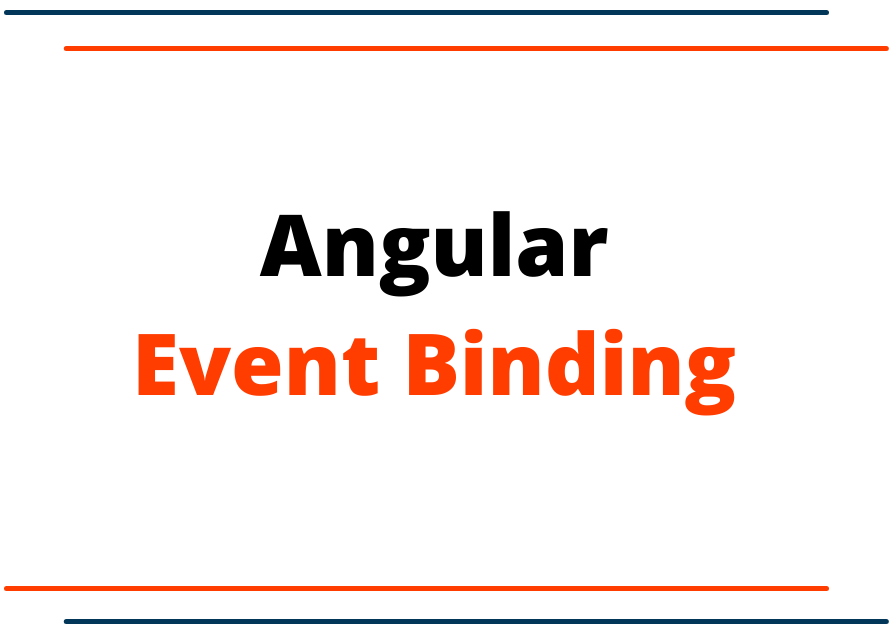
Angular Event Binding
The event binding is also another type of one-way data binding in Angular. But the event binding helps us to pass the data from view to the component. The event could be anything like a mouse click, hover, keystrokes, touch, etc.
Sometimes we need to collect the information from view or from users in that case the event binding plays the role to collect and pass the data to the component so, we can validate the data, save the data to the server, or do any kinds of business logic that we need to do for your application.
Let's see the event binding code syntax.
Let's take a look at an example of the code of Angular event binding.
import { Component } from '@angular/core';
//Define a template within the component withing backticks
@Component({
selector: 'app-demoComponent',
template: `
`
})
export class DemoComponent {
constructor(){
//Constructor
}
doSomething()
{
alert("Hello Angular Developer!");
}
}
In the above example, we have created the doSomething method and when the user clicks on the button, that method get invokes and displays the alert message. But this is a very simple demo. You can do lot more things using the event binding feature that Angular provides us.
Let's see another example of how we can pass the argument to the method from event binding.
import { Component } from '@angular/core';
//Define a template within the component withing backticks
@Component({
selector: 'app-demoComponent',
template: `
`
})
export class DemoComponent {
constructor(){
//Constructor
}
doSomething(event:any)
{
alert(event);
}
}
Notice, that we are passing "Hello Angular Developer!" string message to the doSomething method.
Let's see another quick example to check the list of properties and methods of the event that helps us to do many things by utilizing those information.
import { Component } from '@angular/core';
//Define a template within the component withing backticks
@Component({
selector: 'app-demoComponent',
template: `
`
})
export class DemoComponent {
constructor(){
//Constructor
}
checkSomething(event:any)
{
console.log(event);
}
}
Output:
PointerEvent {isTrusted: true, pointerId: 1, width: 1, height: 1, pressure: 0, …} delegateTarget: document isTrusted: true altKey: false altitudeAngle: 1.5707963267948966 azimuthAngle: 0 bubbles: true button: 0 buttons: 0 cancelBubble: false cancelable: true clientX: 85 clientY: 73 composed: true ctrlKey: false currentTarget: null defaultPrevented: false detail: 1 eventPhase: 0 fromElement: null height: 1 isPrimary: false layerX: 85 layerY: 73 metaKey: false movementX: 0 movementY: 0 offsetX: 37 offsetY: 24 pageX: 85 pageY: 73 path: (8) [button.btn.btn-primary, app-democomponent, div.m-5, app-root, body, html, document, Window] pointerId: 1 pointerType: "mouse" pressure: 0 relatedTarget: null returnValue: true screenX: 85 screenY: 144 shiftKey: false sourceCapabilities: InputDeviceCapabilities {firesTouchEvents: false} srcElement: button.btn.btn-primary tangentialPressure: 0 target: button.btn.btn-primary __ngContext__: LComponentView(22) [app-democomponent, TView, 147, LComponentView(32), null, null, TNode, LCleanup(2), DemoComponent, {…}, DomRendererFactory2, DefaultDomRenderer2, null, null, null, LComponentView(32), LComponentView(22), null, 0, null, button.btn.btn-primary, text, debug: LViewDebug] __zone_symbol__clickfalse: [ZoneTask] accessKey: "" ariaAtomic: null ariaAutoComplete: null ariaBusy: null ariaChecked: null ariaColCount: null ariaColIndex: null ariaColSpan: null ariaCurrent: null ariaDescription: null ariaDisabled: null ariaExpanded: null ariaHasPopup: null ariaHidden: null ariaKeyShortcuts: null ariaLabel: null ariaLevel: null ariaLive: null ariaModal: null ariaMultiLine: null ariaMultiSelectable: null ariaOrientation: null ariaPlaceholder: null ariaPosInSet: null ariaPressed: null ariaReadOnly: null ariaRelevant: null ariaRequired: null ariaRoleDescription: null ariaRowCount: null ariaRowIndex: null ariaRowSpan: null ariaSelected: null ariaSetSize: null ariaSort: null ariaValueMax: null ariaValueMin: null ariaValueNow: null ariaValueText: null assignedSlot: null attributeStyleMap: StylePropertyMap {size: 0} attributes: NamedNodeMap {0: class, class: class, length: 1} autocapitalize: "" autofocus: false baseURI: "http://localhost:4200/" childElementCount: 0 childNodes: NodeList [text] children: HTMLCollection [] classList: DOMTokenList(2) ['btn', 'btn-primary', value: 'btn btn-primary'] className: "btn btn-primary" clientHeight: 36 clientLeft: 1 clientTop: 1 clientWidth: 67 contentEditable: "inherit" dataset: DOMStringMap {} dir: "" disabled: false draggable: false elementTiming: "" enterKeyHint: "" firstChild: text firstElementChild: null form: null formAction: "http://localhost:4200/" formEnctype: "" formMethod: "" formNoValidate: false formTarget: "" hidden: false id: "" innerHTML: "Check" innerText: "Check" inputMode: "" isConnected: true isContentEditable: false labels: NodeList [] lang: "" lastChild: text lastElementChild: null localName: "button" name: "" namespaceURI: "http://www.w3.org/1999/xhtml" nextElementSibling: null nextSibling: null nodeName: "BUTTON" nodeType: 1 nodeValue: null nonce: "" offsetHeight: 38 offsetLeft: 48 offsetParent: body offsetTop: 48 offsetWidth: 69 onabort: (...) onanimationend: (...) onanimationiteration: (...) onanimationstart: (...) onauxclick: (...) onbeforecopy: (...) onbeforecut: (...) onbeforepaste: (...) onbeforexrselect: (...) onblur: (...) oncancel: (...) oncanplay: (...) oncanplaythrough: (...) onchange: (...) onclick: (...) onclose: (...) oncontextlost: (...) oncontextmenu: (...) oncontextrestored: (...) oncopy: (...) oncuechange: (...) oncut: (...) ondblclick: (...) ondrag: (...) ondragend: (...) ondragenter: (...) ondragleave: (...) ondragover: (...) ondragstart: (...) ondrop: (...) ondurationchange: (...) onemptied: (...) onended: (...) onerror: (...) onfocus: (...) onformdata: (...) onfullscreenchange: (...) onfullscreenerror: (...) ongotpointercapture: (...) oninput: (...) oninvalid: (...) onkeydown: (...) onkeypress: (...) onkeyup: (...) onload: (...) onloadeddata: (...) onloadedmetadata: (...) onloadstart: (...) onlostpointercapture: (...) onmousedown: (...) onmouseenter: (...) onmouseleave: (...) onmousemove: (...) onmouseout: (...) onmouseover: (...) onmouseup: (...) onmousewheel: (...) onpaste: (...) onpause: (...) onplay: (...) onplaying: (...) onpointercancel: (...) onpointerdown: (...) onpointerenter: (...) onpointerleave: (...) onpointermove: (...) onpointerout: (...) onpointerover: (...) onpointerrawupdate: (...) onpointerup: (...) onprogress: (...) onratechange: (...) onreset: (...) onresize: (...) onscroll: (...) onsearch: (...) onsecuritypolicyviolation: (...) onseeked: (...) onseeking: (...) onselect: (...) onselectionchange: (...) onselectstart: (...) onslotchange: (...) onstalled: (...) onsubmit: (...) onsuspend: (...) ontimeupdate: (...) ontoggle: (...) ontransitioncancel: (...) ontransitionend: (...) ontransitionrun: (...) ontransitionstart: (...) onvolumechange: (...) onwaiting: (...) onwebkitanimationend: (...) onwebkitanimationiteration: (...) onwebkitanimationstart: (...) onwebkitfullscreenchange: (...) onwebkitfullscreenerror: (...) onwebkittransitionend: (...) onwheel: (...) outerHTML: "" outerText: "Check" ownerDocument: document (...) tiltX: 0 tiltY: 0 timeStamp: 7476.199999928474 toElement: null twist: 0 type: "click" view: Window {window: Window, self: Window, document: document, name: '', location: Location, …} which: 1 width: 1 x: 85 y: 73 [[Prototype]]: PointerEvent