Angular Components
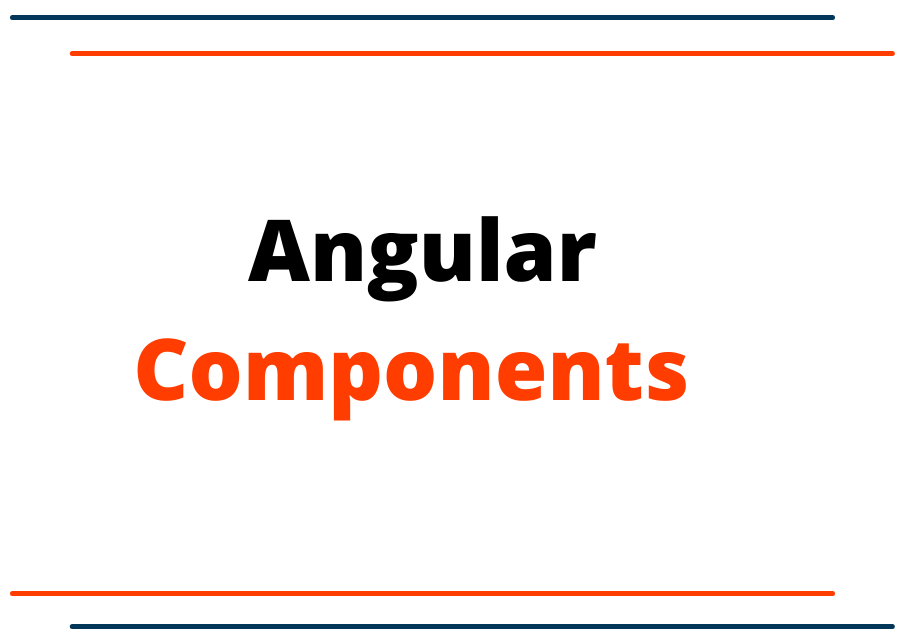
Angular Components
In Angular, the components are the main building block that represents a portion of the view. Components are nothing rather than a combination of HTML, CSS, and JavaScript. There are multiple ways to create the component. Let’s see how to create and use the component in our Angular application.
First, let’s create a component manually by taking the following steps.
- Open the newly created Angular apps in any of your favorite IDE (Integrated Development Environment).
- Once you’ve opened the apps in IDE. Let’s find the src > app folder. Create another folder where the component associate files can be stored. For example, we have created a welcome folder, but you can give any name you want.
- Create a new file inside the welcome folder by following the component creating format component-name.component.ts. So, for example, our component name would be welcome.component.ts.
- After you created the new component file open it in your IDE. At the top of the file add the following import statement.
- After that add the @Compoent decorator to define the HTML, and CSS along with the component name of the selector.
- Finally, add the class that includes the code of the component.
This is what it looks like after you have completed all of the above steps.
welcome.component.ts
welcome.component.html
welcome.component.css
Here is the screenshot of the folder structure.
There is a couple of more steps to take to see how our component renders in the web browser. Let's move on to those steps. First, open the src > app > app.module.ts and include the below code to that file.
Open the src > app > app.component.html and the following code.
Let's open the CLI/Terminal > go to the application folder and run the following highlighted command to build and serve the apps to the browser.
C:\Users\17193\Documents\__DEMO_PROJECTS__\Angular\AngularApp>ng serve -o
The application will be open in default browser.
Well done, you have understood the basics of the Angular component.